面试官系列:讲讲快速失败和安全失败的区别?
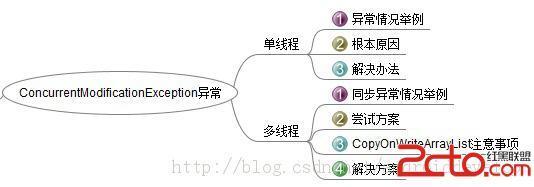
1、问题:Java 的 Fail-fast 和 Safe-fast 有什么区别?
1.1 快速失败 & 安全失败
【快速失败】
在用迭代器遍历一个集合对象时,如果遍历过程中对集合对象的内容进行了修改(增加、删除、修改),则会抛出 Concurrent Modification Exception。
原理:迭代器在遍历时直接访问集合中的内容,并且在遍历过程中使用一个 modCount 变量。
集合在被遍历期间如果内容发生变化,就会改变 modCount 的值。每当迭代器使用 hashNext()/next()遍历下一个元素之前,都会检测 modCount 变量是否为 expectedmodCount 值,是的话就返回遍历;否则抛出异常,终止遍历。
注意:这里异常的抛出条件是检测到 (modCount!=expectedmodCount) 这个条件。如果集合发生变化时修改 modCount 值刚好又设置为了 expectedmodCount 值,则异常不会抛出。因此,不能依赖于这个异常是否抛出而进行并发操作的编程,这个异常只建议用于检测并发修改的 bug。
场景:java.util 包下的集合类都是快速失败的,不能在多线程下发生并发修改(迭代过程中被修改)。
【安全失败】
采用安全失败机制的集合容器,在遍历时不是直接在集合内容上访问的,而是先复制原有集合内容,在拷贝的集合上进行遍历。
原理:由于迭代时是对原集合的拷贝进行遍历,所以在遍历过程中对原集合所作的修改并不能被迭代器检测到,所以不会触发 Concurrent Modification Exception。
缺点:基于拷贝内容的优点是避免了 Concurrent Modification Exception,但同样地,迭代器并不能访问到修改后的内容,即:迭代器遍历的是开始遍历那一刻拿到的集合拷贝,在遍历期间原集合发生的修改迭代器是不知道的。
场景:java.util.concurrent 包下的容器都是安全失败,可以在多线程下并发使用,并发修改。
1.2 ConcurrentModificationException
我们看下 ConcurrentModificationException 源码:
ConcurrentModificationException 属于运行时异常;
注释清楚写明白了:此异常可能由检测到并发的方法引发,因为该对象的修改不被允许。而可能引发这种异常的对象,则可能来源于以下的工具类:Collection,Iterator,Spliterator,ListIterator,Vector,LinkedList,HashSet,Hashtable,TreeMap,AbstractList 等。
2、源码案例
2.1 ArrayList & Vector
ArrayList 本质是一个对象数组,是非线程安全的容器;
而 Vector 则是该容器的线程安全版本,它的线程安全策略是:在 的开放 api 基础上,都加上了互斥锁 -- synchronized,使得对容器的任何操作都串行化执行。
我们都知道,序列化不会自动保存 static 和 transient 变量,因此我们若要保存它们,则需要通过 writeObject()和 readObject()去手动读写,所以通过 writeObject()方法,写入要保存的变量。
2.2 ArrayList 的私有方法 writeObject(快速失败)
我们走读下 ArrayList 的 writeObject(java.io.ObjectOutputStream s) 方法:
2.2.1 代码分析
我们走读这个代码段,在方法的末尾处有个 (modCount != expectedModCount) 判断。条件满足,则抛出了 ConcurrentModificationException 异常。
2.3 Vector 的私有方法
我们走读下 Vector 的 writeObject(java.io.ObjectOutputStream s) 方法:
2.3.1 代码分析
我们走读这个代码段,发现了在将容器数据传递给输出流 ObjectOutputStream 时,使用了 synchronized 锁住了一个代码块。这个代码块的内容呢,就是将容器的数据克隆一份到临时内存,最后写入到输出流;整个过程并不影响原来容器的数据 elementData 的任何属性(只读),因此达到安全失败的要求。
欢迎关注:后台技术汇
博主是一名 Java 后端开发工程师,从业互联网开发已经三年了
目前正在维护一个公众号“后台技术汇”,公众号宗旨是:原创 Java 后台开发技术栈的知识分享,分享程序猿专属干货与福利,希望分享的内容,能给大家工作带来一点帮助。
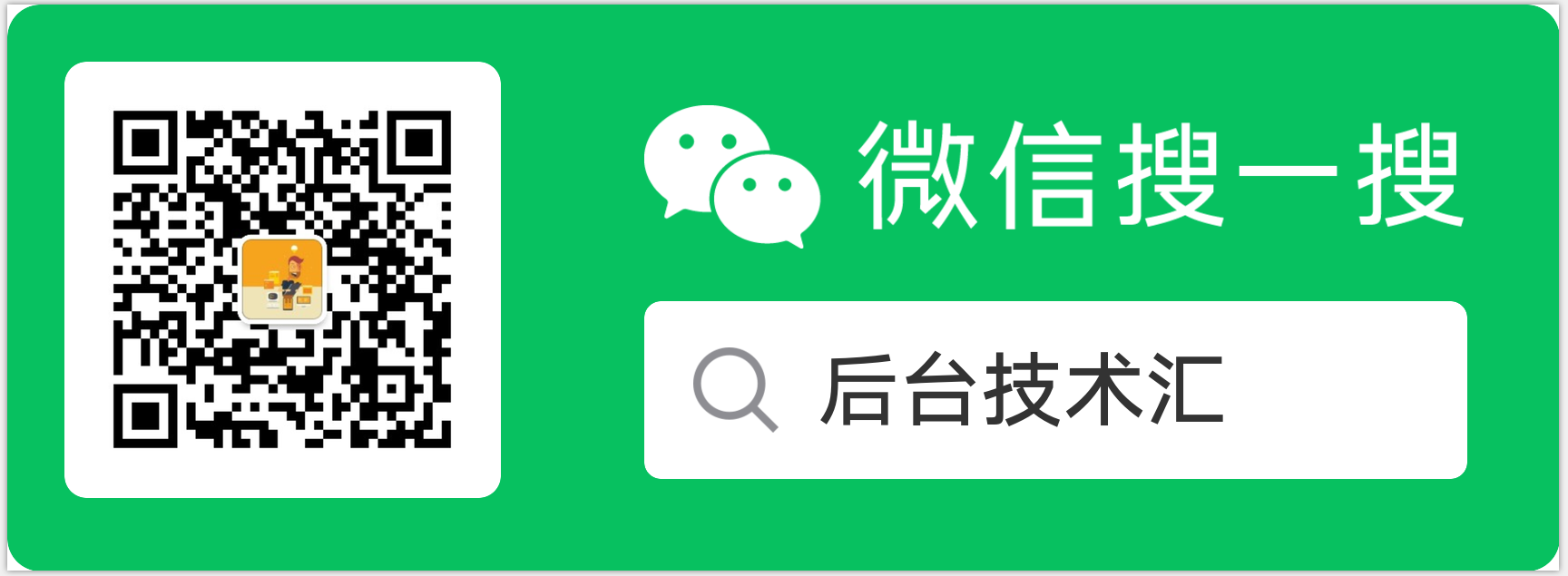
版权声明: 本文为 InfoQ 作者【后台技术汇】的原创文章。
原文链接:【http://xie.infoq.cn/article/46501dfb4e7ee76a378a58a81】。文章转载请联系作者。
评论