第 3 周 - 课后作业
发布于: 2020 年 06 月 23 日
1 请在草稿纸上手写一个单例模式的实现代码。
在草稿纸上手写双重检查锁实现单例模式。
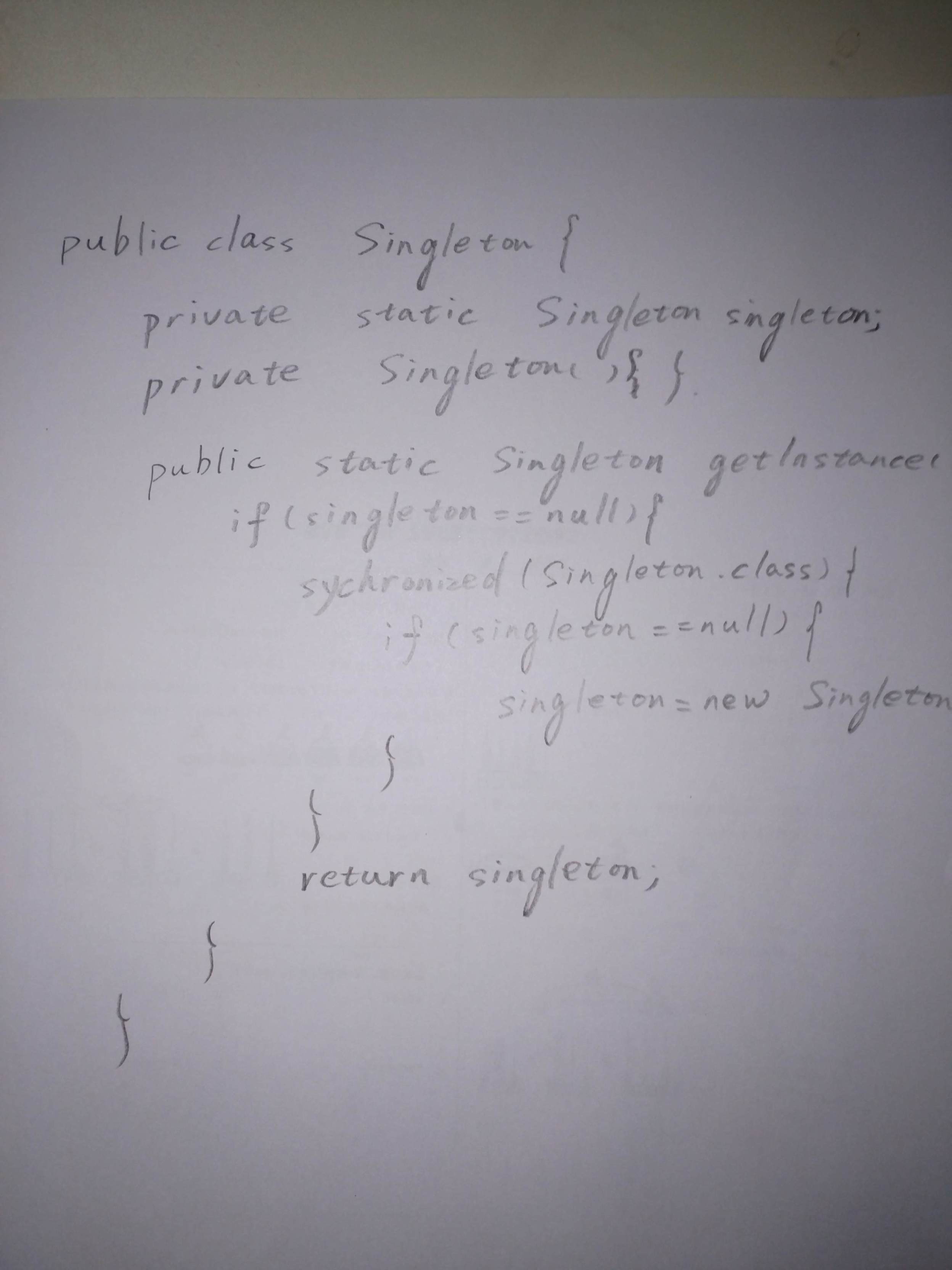
2 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图
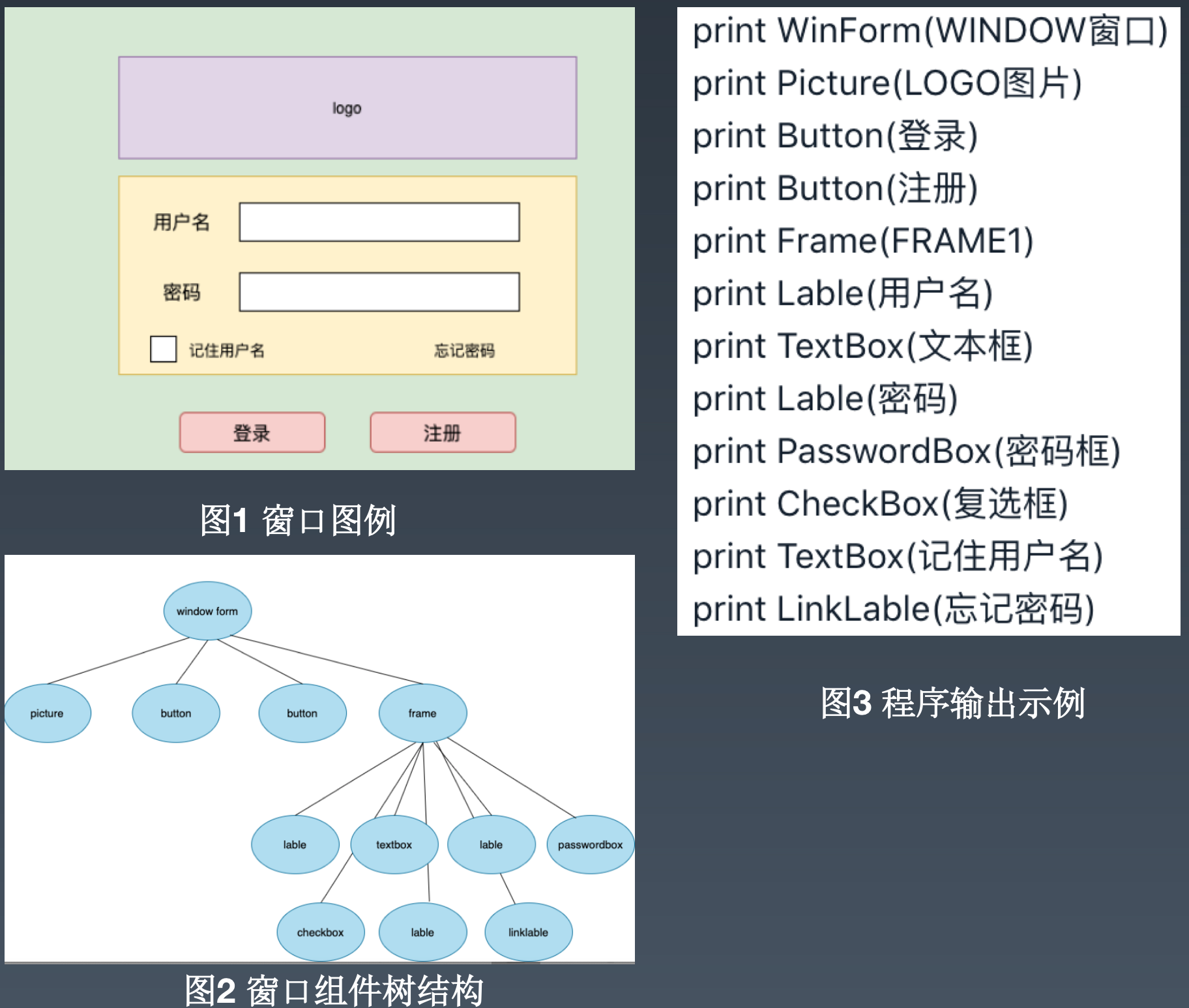
定义一个Module接口
package com.ctec;public interface Module { public void print();}
定义一个含有子组件的抽象类:
package com.ctec;import java.util.ArrayList;import java.util.List;public abstract class CompositeModule implements Module{ private String name; private List<Module> moduleList = new ArrayList<>(); public CompositeModule(String name) { this.name = name; } public CompositeModule add(Module module) { moduleList.add(module); return this; } @Override public void print() { System.out.println(String.format("print %s", name)); for (Module module : moduleList) { module.print(); } }}
package com.ctec;public class WindowForm extends CompositeModule { public WindowForm(String name) { super(name); }}
package com.ctec;public class Button implements Module { private String name; public Button(String name) { this.name = name; } @Override public void print() { System.out.println(String.format("print Button(%s)", name)); }}
package com.ctec;public class Picture implements Module { private String path; public Picture(String path) { this.path = path; } @Override public void print() { System.out.println(String.format("print Picture(%s)", path)); }}
package com.ctec;public class Frame extends CompositeModule { public Frame(String name) { super(name); }}
package com.ctec;public class Label implements Module { private String text; public Label(String text) { this.text = text; } @Override public void print() { System.out.println(String.format("print Label(%s)", text)); }}
package com.ctec;public class TextBox implements Module { private String placeHolder; public TextBox(String placeHolder) { this.placeHolder = placeHolder; } @Override public void print() { System.out.println(String.format("print TextBox(%s)", placeHolder)); }}
package com.ctec;public class PasswordBox implements Module { private String placeHolder; public PasswordBox(String placeHolder) { this.placeHolder = placeHolder; } @Override public void print() { System.out.println(String.format("print TextBox(%s)", placeHolder)); }}
package com.ctec;public class LinkLabel implements Module { private String text; public LinkLabel(String text) { this.text = text; } @Override public void print() { System.out.println(String.format("print LinkLabel(%s)", text)); }}
package com.ctec;public class CheckBox implements Module { @Override public void print() { System.out.println("print CheckBox(复选框)"); }}
测试类
package com.ctec;import org.junit.Test;import static org.junit.Assert.*;public class ModuleTest { @Test public void print() { CompositeModule winForm = new WindowForm("WinForm(WINDOW窗口)"); Module picture = new Picture("LOGO图片"); Module loginButton = new Button("登录"); Module registerButton = new Button("注册"); CompositeModule frame = new Frame("Frame(FRAME1)"); Module usernameLabel = new Label("用户名"); Module usernameTextBox = new TextBox("请输入用户名"); Module passwordLabel = new Label("密码"); Module passwordBox = new PasswordBox("请输入密码"); Module checkbox = new CheckBox(); Module rememberTextBox = new TextBox("记住用户名"); Module linkLabel = new LinkLabel("忘记密码"); frame.add(usernameLabel).add(usernameTextBox).add(passwordLabel).add(passwordBox).add(checkbox).add(rememberTextBox).add(linkLabel); winForm.add(picture).add(loginButton).add(registerButton).add(frame).print(); }}
测试输出结果
print WinForm(WINDOW窗口)print Picture(LOGO图片)print Button(登录)print Button(注册)print Frame(FRAME1)print Label(用户名)print TextBox(请输入用户名)print Label(密码)print TextBox(请输入密码)print CheckBox(复选框)print TextBox(记住用户名)print LinkLabel(忘记密码)
划线
评论
复制
发布于: 2020 年 06 月 23 日阅读数: 56
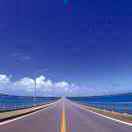
大海
关注
还未添加个人签名 2018.07.14 加入
还未添加个人简介
评论