LeetCode 1396. Design Underground System
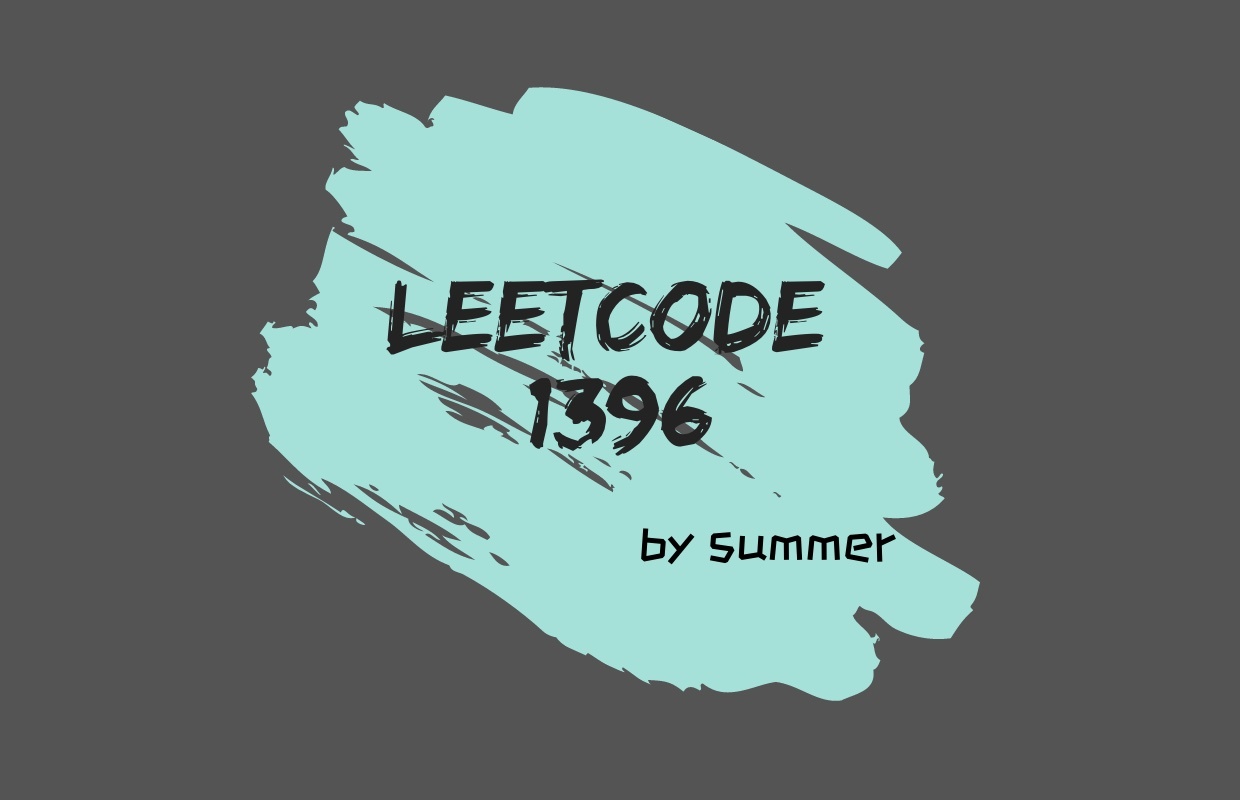
@(LeetCode)
问题描述
实现 UndergroundSystem
类,使其支持如下三个函数:
checkIn(int id, string stationName, int t)
一个卡片
id
为id
的用户,在t
时进站stationName
。一个用户在某个时间点只能进入一个站点。
checkOut(int id, string stationName, int t)
一个卡片
id
为id
的用户,在t
时出站stationName
。
getAverageTime(string startStation, string endStation)
返回出发地为
startStation
,到达地为endStation
的平均旅程时间。平均时间由之前所有从
startStation
直达endStation
的旅程计算而来。对
getAverageTime
的调用总是有效。
你可以假设对 checkIn
和 checkOut
的调用是一致的。也就是说,当一个用户在 t1
时进站,在 t2
时出站,满足 t2 > t1
。所有事件都是按时间顺序发生。
栗 1:
输入:
输出:
解释:
第一次调用
getAverageTime
,此时旅程信息如下:
Paradise-Cambridge
的平均值为:14
。
第二次调用
getAverageTime
,此时旅程信息如下:
Leyton-Waterloo
的平均值为:11 = (12 + 10) / 2
。
第三次调用
getAverageTime
,此时旅程信息如下:
Leyton-Waterloo
的平均值为:11 = (12 + 10) / 2
。
第四次调用
getAverageTime
,此时旅程信息如下:
Leyton-Waterloo
的平均值为:12 = (12 + 10 + 14) / 3
。
注意:
最多有
20000
个操作。1 <= id, t <= 10^6
。所有的字符串包括大小写字母和数字。
1 <= stationName.length <= 10
。答案与实际值相差在
10^-5
以内都是正确的。
想看英文原文的戳这里:https://leetcode.com/problems/design-underground-system。
解题思路
这道题的思路比较简单。由于进站和出站是有先后顺序的,也就是说当出站时,一定有对应的进站信息。那么当调用 checkout
时,便可以计算出某个用户在这段旅程上花费的时间。
而题目要求计算出给定起始点的旅程所花费的平均时间,那么得知道该路线对应的所有旅程信息,即需要记录所有用户在该路线上的旅程时间。而上面我们提到,某个用户在某段旅程上花费的时间是可以计算出来的。
为了快速得到所有旅程信息,能比较容易的想到使用 map
来进行存储。我们只需要将起始点作为 key
,将各用户旅程时间记录下来即可。这是一种一对多的关系,即 { key: [t1, t2] }
。
以上分析对应如下实现:
checkin
, 记录用户id
的进站信息。checkout
,查找用户id
的进站信息,计算旅程时长t
,并将其添加到该路线对应的旅程时间列表中。getAverageTime
,根据起始点,获取路线对应的所有旅程时间列表,计算平均时间。
js
代码如下:
版权声明: 本文为 InfoQ 作者【liu_liu】的原创文章。
原文链接:【http://xie.infoq.cn/article/3cccbe1dd22c92df48de84010】。
本文遵守【CC-BY 4.0】协议,转载请保留原文出处及本版权声明。
评论