TypeScript | 第五章:高级类型
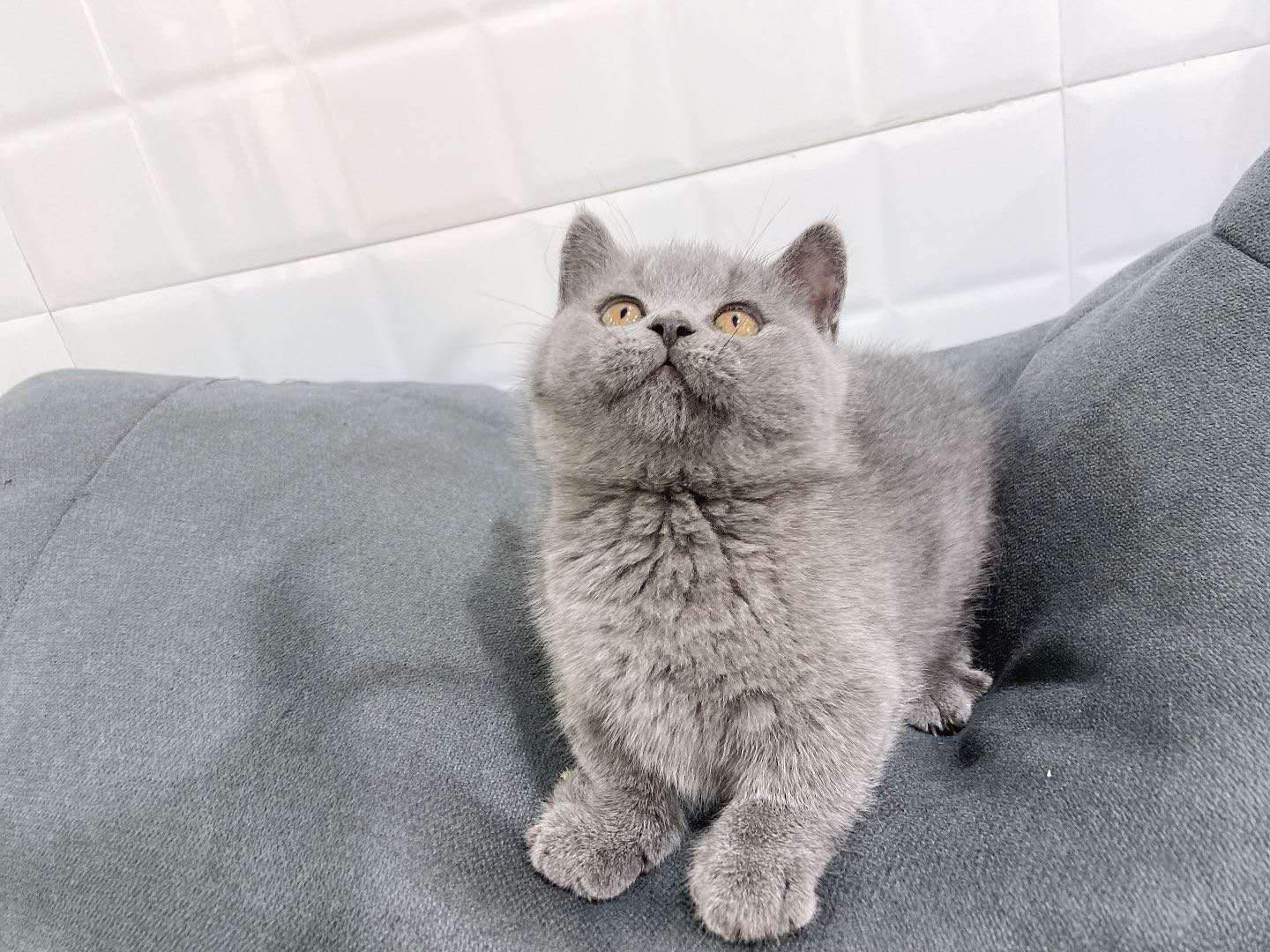
TypeScript系列学习笔记:
TypeScript | 第六章:理解声明合并,以及编写声明文件
1. 交叉类型
交叉类型是将多个类型合并为一个类型,用符号"&"表示。
2. 联合类型
联合类型表示一个值可以是几种类型之一,用符号"|"分隔每个类型。
3. 自动类型推断
1. typeof
TypeScript可以将typeof
识别为一个类型保护,可以直接在代码里检查类型了。
2. instanceof
nstanceof
类型保护是通过构造函数来细化类型的一种方式。
3. 浏览器API
4. 其他方式
4. null 和 undefined
默认情况,类型检查器认为null
与undefined
可以赋值给任何类型,它们是其它类型的一个有效值。
--strictNullChecks
标记可以解决,声明变量时不会自动包含null
或 undefined
。可选参数会自动加上| undefined
。
5. 类型断言
告诉ts这是什么类型,它都信。
6. 类型别名
类型别名会给一个类型起个新名字。 类型别名有时和接口很像,但是可以作用于原始值,联合类型,元组以及其它任何你需要手写的类型。
型别名可以表示很多接口表示不了的类型, 比如字面量类型(常用来校验取值范围)。
类型别名不能出现在声明右侧的任何地方。
7. 索引类型
ts中的keyof
和他类似, 可以用来获取对象类型的键值。
8. 映射类型
映射类型比较像修改类型的工具函数。
infer:待推断/延迟推断,根据实际情况推断类型。
1. Readonly
2. Partial<T>
3. Required<T>
4. Pick<T,K>
5. Omit<T,K>
6. Record<K,T>
7. Exclude<T,U>
8. Extract<T,U>
9. NonNullable
9. ReturnType
10. InstanceType
返回T的实例类型。
ts中类有2种类型, 静态部分的类型和实例的类型, 所以T
如果是构造函数类型, 那么InstanceType
可以返回他的实例类型:
11. Parameters<T>
12. ConstructorParameters<T>
9. extends
10. 总结
至此完成了高级类型的学习,不完善的地方后续陆续补充。
版权声明: 本文为 InfoQ 作者【梁龙先森】的原创文章。
原文链接:【http://xie.infoq.cn/article/38206f29d1a77a62cf0517a81】。文章转载请联系作者。
评论