架构师训练营第三周作业
发布于: 2020 年 10 月 04 日
作业一
1、
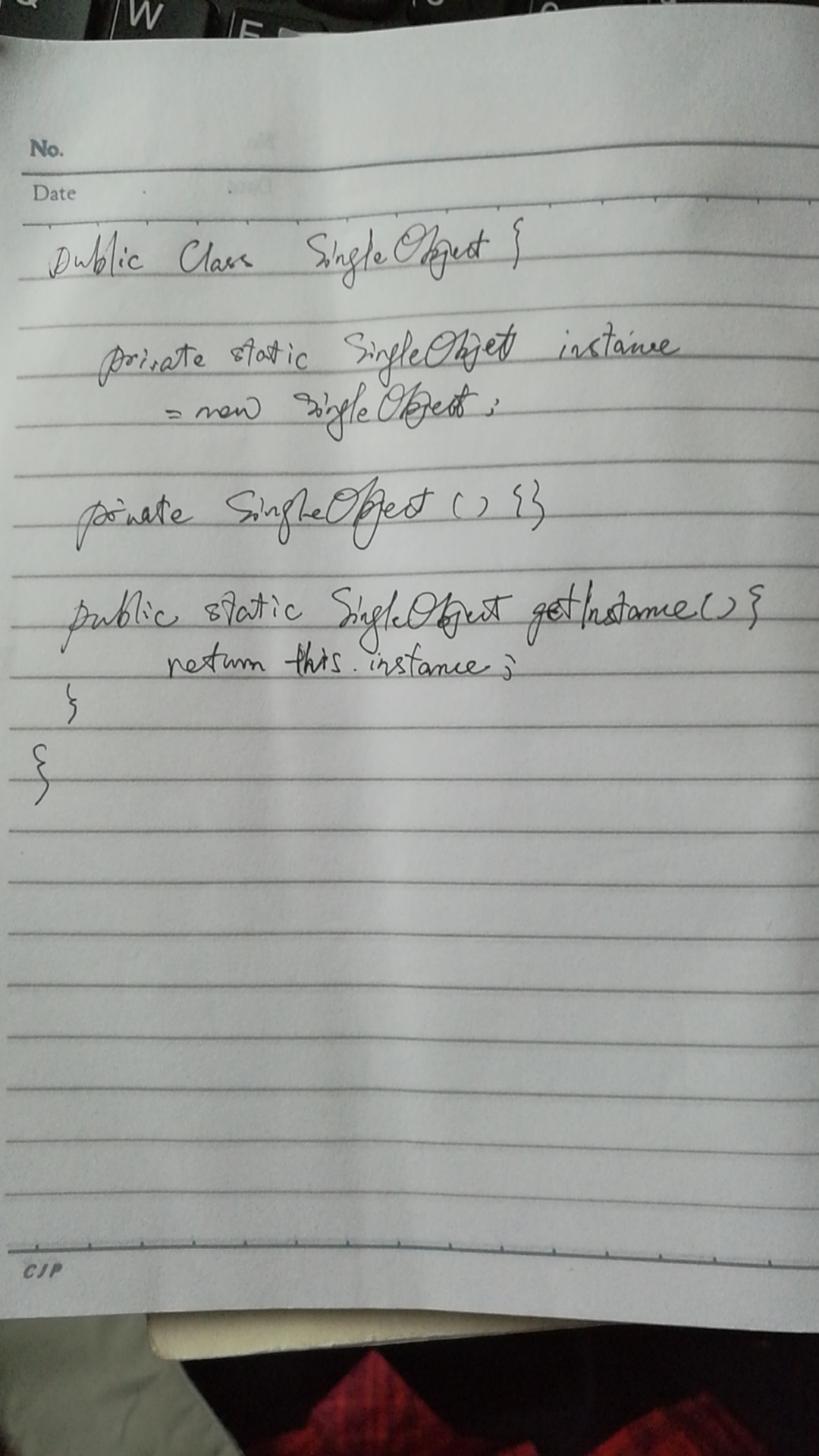
2、
import java.util.ArrayList;
public abstract class Component {
protected String name;
protected ArrayList<Component> lstComponets;
public Component(String name) {
this.name = name;
}
public void add(Component component){
this.lstComponets.add(component);
}
public void remove(Component component) {
this.lstComponets.remove(component);
}
public abstract void print();
}
public class Winform extends Component {
public Winform(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print WinForm("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class Picture extends Component {
public Picture(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print WinForm("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class Button extends Component {
public Button(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print Button("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class Frame extends Component {
public Frame(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print Frame("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class Label extends Component {
public Label(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print Label("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class TextBox extends Component {
public TextBox(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print TextBox("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class PasswordBox extends Component {
public PasswordBox(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print PasswordBox("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class CheckBox extends Component {
public CheckBox(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print CheckBox("+this.name+")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class LinkLabel extends Component {
public LinkLabel(String name) {
super(name);
}
@Override
public void print() {
System.out.println("print LinkLabel(" + this.name + ")");
for (Component child : lstComponets) {
child.print();
}
}
}
public class Client {
public static void main(String[] args) {
Component winform = new Winform("WINDOWS窗口");
winform.add(new Picture("LOGO"));
Component frame = new Frame("FRAME");
frame.add(new Label("用户名"));
frame.add(new TextBox("文本框"));
frame.add(new Label("密码"));
frame.add(new PasswordBox("密码框"));
frame.add(new CheckBox("复选框"));
frame.add(new TextBox("记住用户名"));
frame.add(new LinkLabel("忘记密码"));
winform.add(frame);
winform.add(new Button("登录"));
winform.add(new Button("注册"));
winform.print();
}
}
复制代码
作业二
本周主要对常用设计模式结合实例进行了学习。之前虽然使用面向对象语言,但任然面向过程的写法较多,每个类只是对相关的方法进行了归集,每个方法有一长串的参数以及一堆的 ifelse,导致需求变更时不断的修改代码。经过一段时间后,不但接手的人,包括自己都无法看懂代码和修改代码。也经常出现牵一发动全身的情况,bug 不断。本周的设计模式也是对第二周设计原则的一个很好的实践。下步需要逐步重构自己之前的代码。
一个设计模式需要记住的四个部分:
模式的名称 - 由少量的字组成的名称,有助于我们表达我们的设计。
待解问题 - 描述了何时需要运用这种模式,以及运用模式的环境(上下文)。
解决方案 - 描述了组成设计的元素(类和对象)、它们的关系、职责以及合作。但这种解
决方案是抽象的,它不代表具体的实现。
结论 - 运用这种方案所带来的利和弊。主要是指它对系统的弹性、扩展性、和可移植性的
影响。
工厂模式:创建类似但不同对象
单例模式:全系统就一个对象
适配器模式:将一个接口转换为另一个接口
装饰器模式:自己包装自己,可以用接口、抽象类,甚至是一个普通的类也可以实现
模板模式:按固定的过程实现
策略模式:按条件实现不同的方法
划线
评论
复制
发布于: 2020 年 10 月 04 日阅读数: 40
我是谁
关注
还未添加个人签名 2017.12.04 加入
十五年电子政务老兵
评论