week03 作业
发布于: 2020 年 06 月 24 日
一个单例模式的实现代码(手写):

组合模式练习:
组合模式是一种”对象的结构模式“。
组合模式(Composite Pattern)将对象组合成树形结构以表示“部分-整体”的层次结构。组合模式使得用户可以使用一致的方法操作单个对象和组合对象。
组合关系与聚合关系的区别:
(1)组合关系:在古代皇帝三宫六院,贵妃很多,但是每一个贵妃只属于皇帝。
(2)聚合关系:一个老师有很多学生,但是每一个学生又属于多个老师。
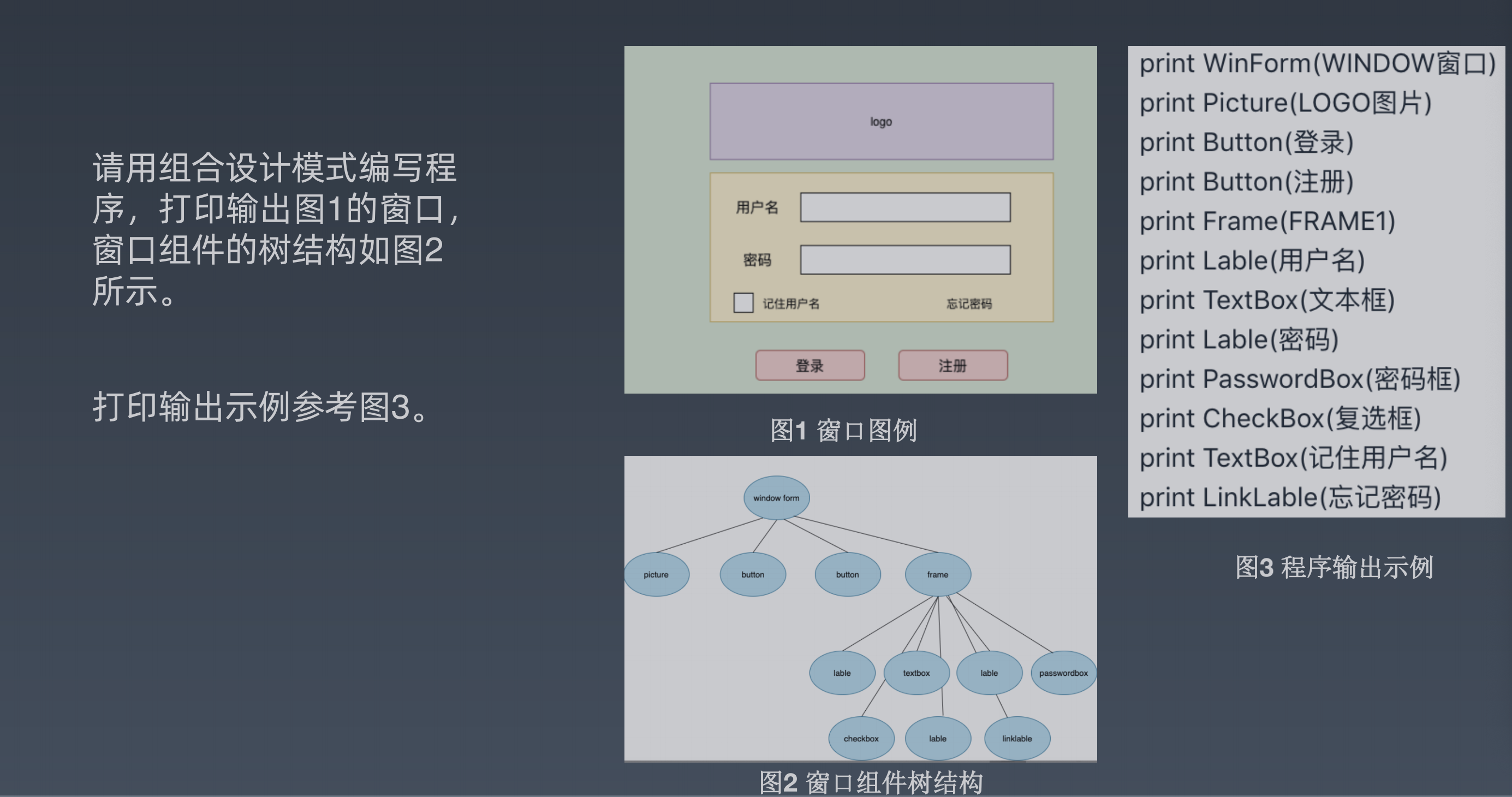
代码实现如下:
import java.util.ArrayList;import java.util.List;public class WinForm implements IWinComponent{ /** 名称 **/ private String name; /** 宽度 **/ private int width; /** 高度 **/ private int heigth; /** 背景色 **/ private String background; private List<WinForm> components; public WinForm(String name) { this.name = name; } public void addComponents(WinForm component) { if(components == null){ components = new ArrayList<>(); } components.add(component); } @Override public void onClick() { System.out.println("这是一个窗口"); } @Override public void draw() { System.out.println("开始绘制一个窗口"); for(WinForm component : components){ component.draw(); } System.out.println("窗口绘制结束"); } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getWidth() { return width; } public void setWidth(int width) { this.width = width; } public int getHeigth() { return heigth; } public void setHeigth(int heigth) { this.heigth = heigth; } public String getBackground() { return background; } public void setBackground(String background) { this.background = background; }}
public class Picture extends WinForm{ private String src; public Picture(String name, String src) { super(name); this.src = src; } @Override public void draw() { System.out.println("画一个logo,图片地址:" + src); }}
public class Button extends WinForm{ private String text; public Button(String name, String text) { super(name); this.text = text; } @Override public void draw() { System.out.println("画一个" + text + "按钮"); }}
import java.util.ArrayList;import java.util.List;public class Frame extends WinForm{ private List<Frame> components; public Frame(String name) { super(name); } public void addComponents(Frame component) { if(components == null){ components = new ArrayList<>(); } components.add(component); } @Override public void draw() { System.out.println("开始绘制一个Frame面板"); for(Frame component : components){ component.draw(); } System.out.println("Frame绘制结束"); }}
public class Lable extends Frame{ private String text; public Lable(String name, String text) { super(name); this.text = text; } @Override public void draw() { System.out.println("画一个标签,文字内容:" + text); }}
public class TextBox extends Frame{ private String input; public TextBox(String name) { super(name); } @Override public void draw() { System.out.println("画一个输入框"); }}
public class PasswordBox extends Frame{ private String password; public PasswordBox(String name) { super(name); } @Override public void draw() { System.out.println("画一个密码框"); }}
public class CheckBox extends Frame{ private String text; public CheckBox(String name, String text) { super(name); this.text = text; } @Override public void draw() { System.out.println("画一个复选框,文本内容:" + text); }}
public class LinkLable extends Frame{ private String text; private String link; public LinkLable(String name, String text, String link) { super(name); this.text = text; this.link = link; } @Override public void draw() { System.out.println("画一个链接标签,文字内容:" + text + ",链接:" + link); }}
public class Test { public static void main(String[] args) { WinForm winform = new WinForm("WINDOW窗口"); Picture picture = new Picture("logo图片", "xxxx/xxx.png"); Button loginBtn = new Button("按钮-1","登录"); Button registerBtn = new Button("按钮-2","注册"); Frame frame = new Frame("FRAME1"); winform.addComponents(picture); winform.addComponents(loginBtn); winform.addComponents(registerBtn); winform.addComponents(frame); Lable lable1 = new Lable("标签1","用户名"); TextBox textBox = new TextBox("文本框"); Lable lable2 = new Lable("标签2","密码"); PasswordBox passwordBox = new PasswordBox("密码框"); CheckBox checkBox = new CheckBox("复选框", "记住用户名"); LinkLable linkLable = new LinkLable("链接标签", "忘记密码", "xxxx/xxx.html"); frame.addComponents(lable1); frame.addComponents(textBox); frame.addComponents(lable2); frame.addComponents(passwordBox); frame.addComponents(checkBox); frame.addComponents(linkLable); winform.draw(); }}
public interface IWinComponent { void onClick(); void draw();}
划线
评论
复制
发布于: 2020 年 06 月 24 日阅读数: 51
Geek_196d0f
关注
还未添加个人签名 2018.09.06 加入
还未添加个人简介
评论