架构师训练营 - 第三周作业
作业一:
1. 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
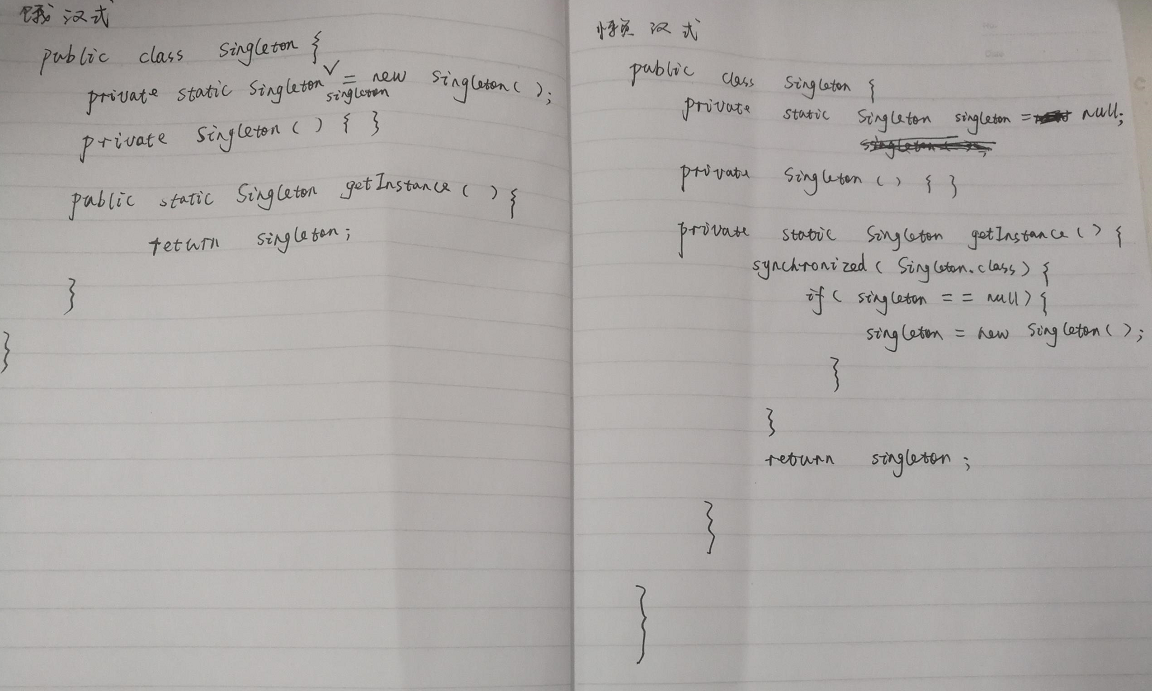
饿汉式:类加载时就实例化对象,使用时直接返回
懒汉式:类加载时先不实例化,使用时再实例化,为了防止多线程问题,方法内加入类同步锁
2. 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
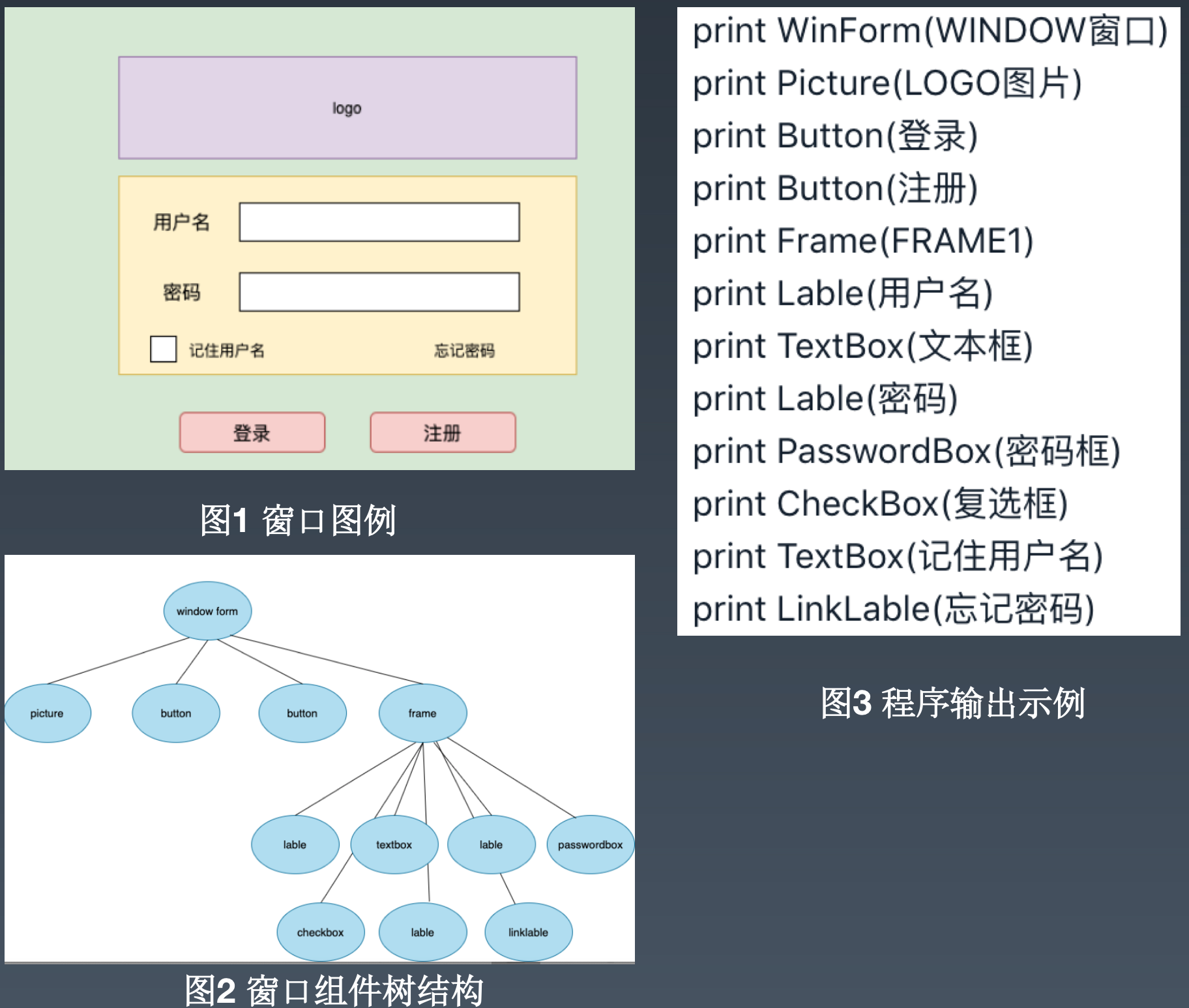
组合模式的定义:
将一组对象(员工和部门)组织成树形结构,以表示一种‘部分 - 整体’的层次结构(部门与子部门的嵌套结构)。组合模式让客户端可以统一单个对象(员工)和组合对象(部门)的处理逻辑(递归遍历)。
从图2-窗口组件树形结构得知,在所有组件中,只有WinForm和Frame包含子组件,所有组件都有共同的打印自身的逻辑,WinForm和Frame除了打印自身外,还需要遍历打印子组件信息。因此,根据组合模式,可以定义一个包含打印定义方法的组件抽象基类。
类设计如下:
定义组件抽象类Component,统一定义打印方法
WinForm类
Picture类
Button类
Frame类
Label类
TextBox类
PasswordBox类
CheckBox类
LinkLable类
Main类
运行结果:
print WinForm(WINDOW窗口)
print Picture(LOGO图片)
print Button(登录)
print Button(注册)
print Frame(FRAME1)
print Label(用户名)
print TextBox(文本框)
print Label(密码)
print PasswordBox(密码框)
print CheckBox(复选框)
print TextBox(记住用户名)
print LinkLable(忘记密码)
评论