1
第七周
发布于: 2020 年 07 月 22 日
1、性能压测的时候,随着并发压力的增加,系统响应时间和吞吐量如何变化,为什么?
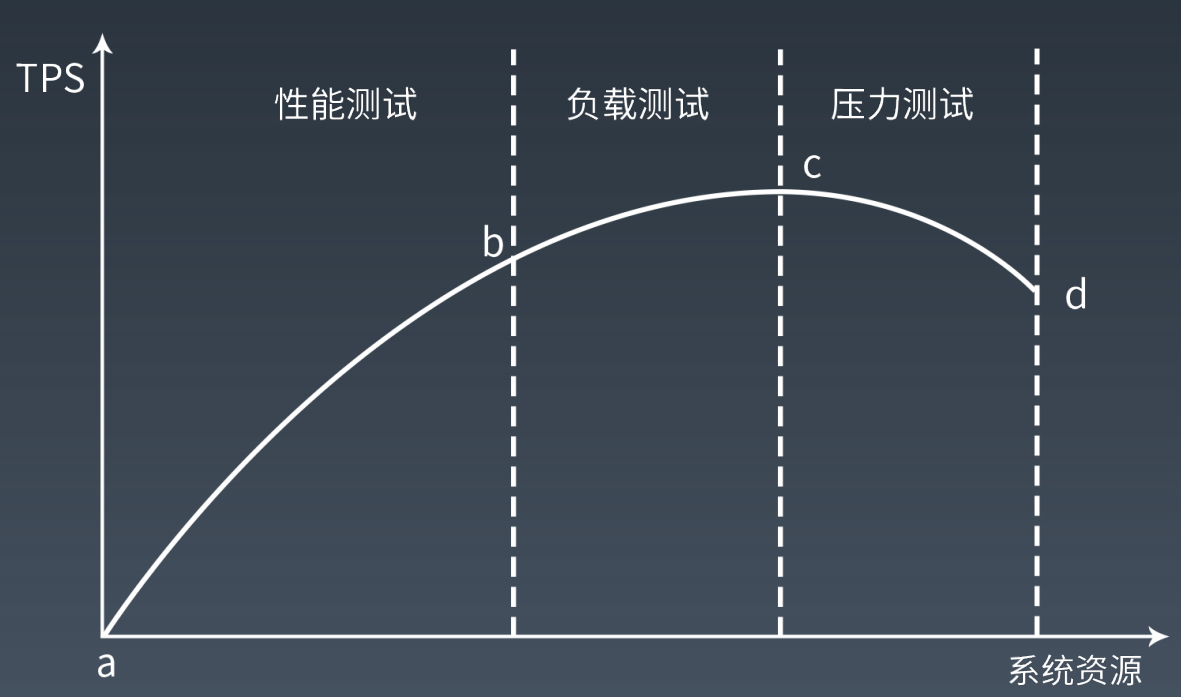
随着并发压力的增加,系统的吞吐逐步增加,此时应该逐步达到b点,即我们期望的性能;再增加压力,某项资源达到临界值,如CPU、内存、IO等,此时吞吐量将再升高之后到达C点,不再变化;如果并发再次增加,系统即超过负载,崩溃,不再可用,此时吞吐为0。
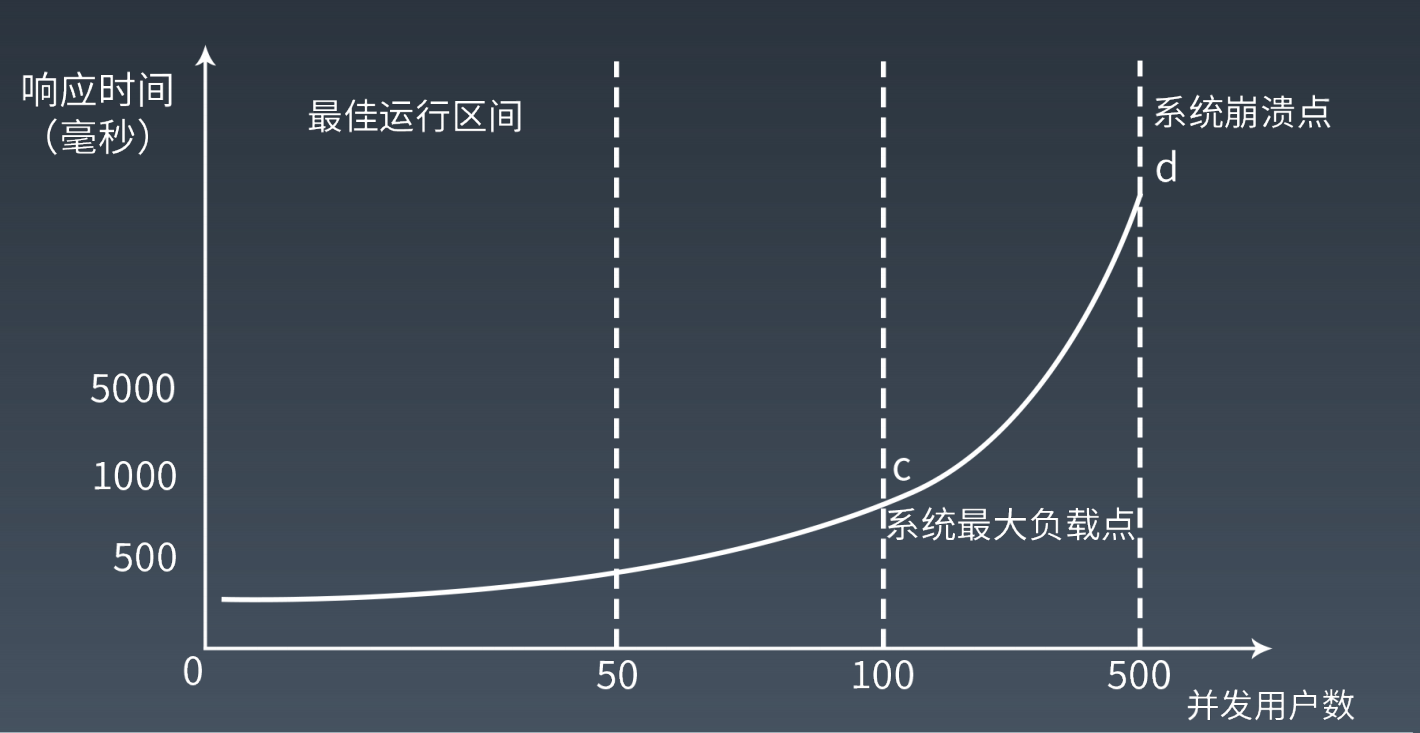
随着并发压力的增加,由于系统的资源是充足的,因此响应时间不会有明显的变化;当到达某一点的时候,由于某项资源到达临界值,系统响应时间快速增长;随着并发压力的再次上升,系统崩溃,不可用。
2、用你熟悉的编程语言写一个web性能压测工具,输入参数:URL,请求总次数,并发数。输出参数:平均响应时间,95%响应时间。用这个测试工具以10并发,100次请求压测www.baidu.com。
package com.leopo.capacitytestutils;import java.io.IOException;import java.io.InputStream;import java.net.HttpURLConnection;import java.net.URL;import java.util.ArrayList;import java.util.Collections;import java.util.List;import java.util.Queue;import java.util.concurrent.ConcurrentLinkedDeque;import java.util.concurrent.CountDownLatch;import java.util.concurrent.ExecutorService;import java.util.concurrent.Executors;public class CapacityTestingTool { /** * URL */ private String url; /** * 请求数 */ private int sumRequest; /** * 线程池 */ private ExecutorService pool; public CapacityTestingTool(String url,int sumRequest,ExecutorService pool){ this.url = url; this.sumRequest = sumRequest; this.pool = pool; } /** * 执行的核心方法 * @return */ public double[] run(){ //用于计数和调度 CountDownLatch doneSignal = new CountDownLatch(sumRequest); //保存每个线程的执行时间,线程安全 Queue<Long> timeQueue = new ConcurrentLinkedDeque<>(); /* *创建100个线程 */ for(int i = 0; i < sumRequest;i++){ pool.execute(new Runnable() { @Override public void run() { HttpURLConnection con = null; long startTime = System.currentTimeMillis(); try { URL link = new URL(url); con = (HttpURLConnection) link.openConnection(); con.setRequestMethod("GET"); InputStream response = con.getInputStream(); //读流,为了减少测试误差,次数省略读流的操作 response.close(); } catch (IOException e) { e.printStackTrace(); } finally { if (con != null) { con.disconnect(); } } long endTime = System.currentTimeMillis(); timeQueue.add(endTime - startTime); doneSignal.countDown(); } }); } try { doneSignal.await(); } catch (InterruptedException e) { e.printStackTrace(); } /* *处理返回平均时间和95%的时间 */ List<Long> times = new ArrayList<>(timeQueue); double avgResponseTime = getAvg(times) / 1000.0; double ninetyFivePercentileTime = get95Percentile(times) / 1000.0; return new double[]{avgResponseTime, ninetyFivePercentileTime}; } private static double getAvg(List<Long> times) { long total = 0; for (long time : times) { total += time; } return total / (float)times.size(); } private static long get95Percentile(List<Long> times) { Collections.sort(times); int index = (int) Math.ceil(95 / 100.0 * times.size()); return times.get(index-1); } public static void main(String[] args) { ExecutorService pool = Executors.newFixedThreadPool(10); CapacityTestingTool tool = new CapacityTestingTool("https://www.baidu.com", 100, pool); double[] metrics = tool.run(); System.out.println("The average response time is " + metrics[0] + " \r\nseconds and the 95 " + "percentile response time is " + metrics[1] + " seconds"); pool.shutdown(); }}
结果是:
The average response time is 0.08411000061035157 seconds and the 95 percentile response time is 0.69 seconds
划线
评论
复制
发布于: 2020 年 07 月 22 日阅读数: 48
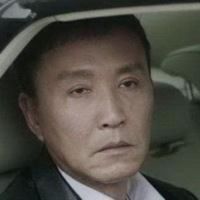
路人
关注
还未添加个人签名 2018.07.26 加入
还未添加个人简介
评论