ARTS - Week Two
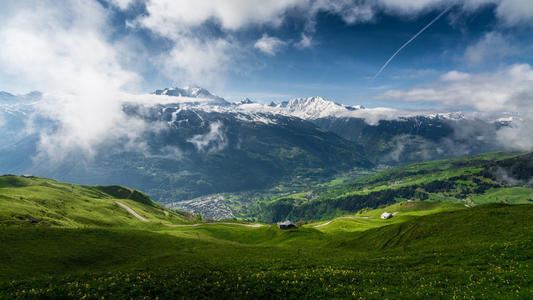
Algorithm
Problem
Binary Tree Preorder Traversal
Given a binary tree, return the preorder traversal of its nodes' values.
Solution
Review
Artical
How to Read an Academic Article
Link
https://organizationsandmarkets.com/2010/08/31/how-to-read-an-academic-article/
Review
An academic article may very hard to be read, and it may also not useful to us. So, for saving our time, we need some tips when we want te get some informations from an aticle by read it. There are some advices from the author who write this article:
1.Klein’s basic steps for skimming, scanning, processing…
Read the abstract (if provided)
Read the introduction
Read the conclusion
Skim the middle, looking at section titles, tables, figures, etc.—try to get a feel for the style and flow of the article.
Is it methodological, conceptual, theoretical (verbal or mathematical), empirical, or something else?
Is it primarily a survey, a novel theoretical contribution, an empirical application of an existing theory or technique, a critique, or something else?
Go back and read the whole thing quickly, skipping equations, most figures and tables.
Go back and read the whole thing carefully, focusing on the sections or areas that seem most important.
2.Once you’ve grasped the basic argument the author is trying to make, critique it!
Ask if the argument makes sense. Is it internally consistent? Well supported by argument or evidence? (This skill takes some experience to develop!)
Compare the article to others you’ve read on the same or a closely related subject. (If this is the first paper you’ve read in a particular subject area, find some more and skim them. Introductions and conclusions are key.) Compare and contrast. Are the arguments consistent, contradictory, orthogonal?
Use Google Scholar, the Social Sciences Citation Index, publisher web pages, and other resources to find articles that cite the article you’re reading. See what they say about it. See if it’s mentioned on blogs, groups, etc.
Check out a reference work, e.g. a survey article from the Journal of Economic Literature, a Handbook or Encyclopedia article, or a similar source, to see how this article fits in the broader context of its subject area.
Actually, I almost copy the whole article to the above part.
Tips
Have you ever use TS + VUE + Element-UI as a frame to develop your project? Here is a pit I encountered:
I hope I have made it clear with my poor English.
Share
Artical
Glossary of Modern JavaScript Concepts: Part 2
Link
https://auth0.com/blog/glossary-of-modern-javascript-concepts-part-2/
Summary
1.Scope (Global, Local, Lexical) and Closures
A closure is formed when a function (alertGreeting
) declared inside an outer function (whenMeetingJohn
) references variables from the outer function's local scope (such as the greeting
variable).
The term "closure" refers to the function and the lexical environment (any local variables that were in scope when the closure was created) in which that function was declared.
2.One-Way Data Flow and Two-Way Data Binding
An application or framework with one-way data flow uses the model as the single source of truth. React is a widely recognized example of one-way data flow (or one-way data binding). Messages are sent from the UI in the form of events to signal the model to update.
In two-way data binding, the data flows in both directions. This means that the JS can update the model and the UI can do so as well. A common example of two-way data binding is with AngularJS.
3.Change Detection in JS Frameworks: Dirty Checking, Accessors, Virtual DOM
Dirty checking refers to a deep comparison that is run on all models in the view to check for a changed value. AngularJS's digest cycle adds a watcher for every property we add to the
$scope
and bind in the UI. Another watcher is added when we want to watch values for changes using$scope.$watch()
.Ember and Backbone use data accessors (getters and setters) for change detection. Ember objects inherit from Ember's APIs and have get() and set() methods that must be used to update models with data binding. This enables the binding between the UI and the data model and Ember then knows exactly what changed. In turn, only the modified data triggers change events to update the app.
Virtual DOM is used by React (and Inferno.js) to implement change detection. React doesn't specifically detect each change. Instead, the virtual DOM is used to diff the previous state of the UI and the new state when a change occurs. React is notified of such changes by the use of the setState() method, which triggers the render() method to perform a diff.
4.Web Components
Web components are encapsulated, reusable widgets based on web platform APIs. They are composed of four standards:
Web components allow us to architect and import custom elements that automatically associate JS behavior with templates and can utilize shadow DOM to provide CSS scoping and DOM encapsulation.
5.Smart and Dumb Components
Smart Components: Also known as container components, smart components can manage interactions with the application's state and data. They handle business logic and respond to events emitted from children (which are often dumb components).
Focus on "How things work"
Provide data and behavior to other components
Usually have no or very little DOM markup, and never have styles
Are often stateful data sources
Are generally generated from higher order components
Dumb Components: Also known as presentational components, dumb components rely on inputs supplied by their parents and are ignorant of application state. They can be sometimes be considered pure and are modular and reusable. They can communicate to their parents when reacting to an event, but they don't handle the event themselves.
Focus on "How things look"
Allow containment with
this.props.children
No dependencies on the rest of the app (ie., no Flux or Redux actions or stores)
Only receive data; do not load or mutate it
Are generally functional (with exceptions)
6.JIT (Just-In-Time) Compilation
Just-In-time (JIT) compilation is the process of translating code written in a programming language to machine code at runtime (during a program or application's execution). At runtime, certain dynamic information is available, such as type identification. A JIT compiler monitors to detect functions or loops of code that are run multiple times—this code is considered "warm". These pieces of code are then compiled. If they're quite commonly executed ("hot"), JIT will optimize them and also store the optimized, compiled code for execution.
7.AOT (Ahead-Of-Time) Compilation
Ahead-Of-Time (AOT) compilation is the process of translating code written in a programming language to machine code before execution (as opposed to at runtime). Doing so reduces runtime overhead and compiles all files together rather than separately.
There are several benefits to AOT for production builds:
Fewer asynchronous requests: templates and styles are inlined with JS
Smaller download size: the compiler doesn't need to be downloaded if the app is already compiled
Detect template errors earlier: compiler detects binding errors during build rather than at runtime
Better security: evaluation is already done, which lowers chance of injection
8.Tree Shaking
Tree shaking is a JavaScript module bundling term that refers to the static analysis of all imported code and exclusion of anything that isn't actually used.
版权声明: 本文为 InfoQ 作者【shepherd】的原创文章。
原文链接:【http://xie.infoq.cn/article/2a386991512c37bcd15731b47】。文章转载请联系作者。
评论