设计模式 -- 组合模式 / 装饰模式
1 JUnit中的设计模式(下)
1.1 参数化的单元测试
public abstract class ComparatorTests<T> extends TestCase{ //对T类型数据比较测试
protected T o1;
protected T o2;
protected boolean ascending;
protected boolean isBefore;
public ComparatorTests(T o1,T o2,boolean ascending,boolean isBefore){
super("testIsBefore");
this.o1=o1;
this.o2=o2;
this.ascending=ascending;
this.isBefore=isBefore;
}
public void testIsBefore(){
assertEquals(isBefore,createComparator(ascending).isBefore(o1,o2));
}
protected abstract Comparator<T> createComparator(boolean ascending);
}
1.2 Integer比较器测试
public class IntegerComparatorTests extends ComparatorTests<Integer>{
public static Test suite(){
TestSuite suite=new TestSuite("IntegerComparatorTests");
suite.addTest(new IntegerComparatorTests(1,1,true,false));
suite.addTest(new IntegerComparatorTests(1,2,true,true));
suite.addTest(new IntegerComparatorTests(2,1,true,false));
suite.addTest(new IntegerComparatorTests(1,1,false,false));
suite.addTest(new IntegerComparatorTests(1,2,false,false));
suite.addTest(new IntegerComparatorTests(2,1,false,true));
return true;
}
public IntegerComparatorTests(Integer o1,integer o2,boolean ascending,boolean isBefore){
super(o1,o2,ascending,isBefore);
}
@Override
protected Comparator<Integer> createComparator(boolean ascending){
return new IntegerComparator(ascending);
}
}
1.3 生成更复杂的“测试包”
public class AllTests{
public static Test suite(){ //测试套件添加其他测试套件和测试用例,形成更复杂的测试包,通过组合模式,生成测试包
TestSuite suite=new TestSuite("sort");
suite.addTestSuite(BubbleSorterTests.class);
suite.addTestSuite(InsertionSorterTests.class);
suite.addTestSuite(ArraySortableTests.class);
suite.addTestSuite(ListSortableTests.class);
suite.addTest(IntegerComparatorTests.suite());
suite.addTest(ComparableComparatorTests.suite());
return suite;
}
}
2 组合模式(Composite)
2.1组合模式--树结构
------是一种“对象的结构模式”:树状结构:叶子节点和组合节点,使用同一方式进行处理。
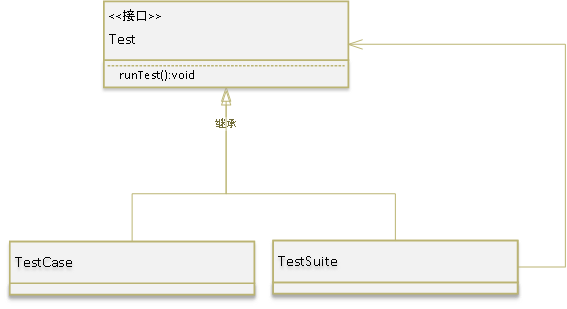
2.2组合模式的应用:
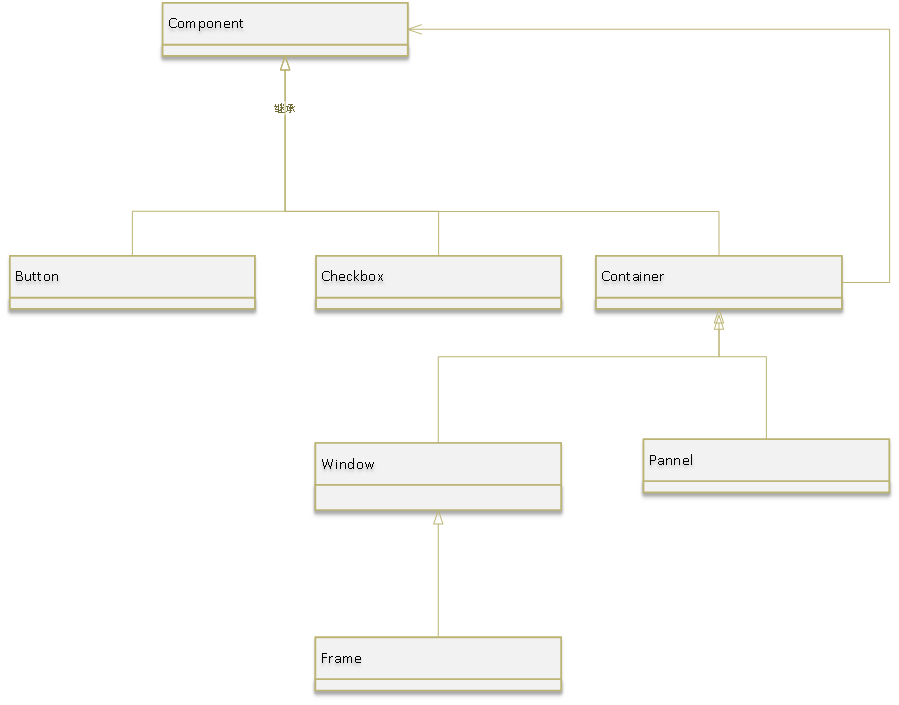
测试排序程序的性能:
冒泡排序和插入排序,谁更快?
重复多次(比如:10000)才能准确的计算出性能
如何让BubbleSorterTests和InsertionSorterTests重复运行多次,而不需要修改代码
如何计算时间?
运用Junit扩展包中的辅助类:
junit.extensions.TestSetup
junit.extensions.RepeatedSetup
2.3性能测试程序:
public class PerformanceTests extends TestSetup{
private long start;
private int repeat;
public PerformanceTests(Test test,int repeat){
super(new RepeatedTest(test,repeat));
this.repeat=repeat;
}
protected void setUp()throws Exception{
start=System.currentTimeMillis();
}
protected void tearDown()throws Exception{
long duration=System.currentTimeMillis()-start;
System.out.printf("%s repeated %d times,takes %d ms\n",getTest(),repeat,duration);
}
public static Test suite(){
TestSuite suite=new TestSuite("performance");
Test bubbleTests=new TestSuite(BubbleSorterTests.class);
Test insertionTests=new TestSuite(InsertionSortsTest.class);
suite.addTest(new PerformanceTests(bubbleTests,10000));
suite.addTest(new PerformanceTests(insertionTests.10000));
return suite;
}
}
3 装饰器模式(Decorator):
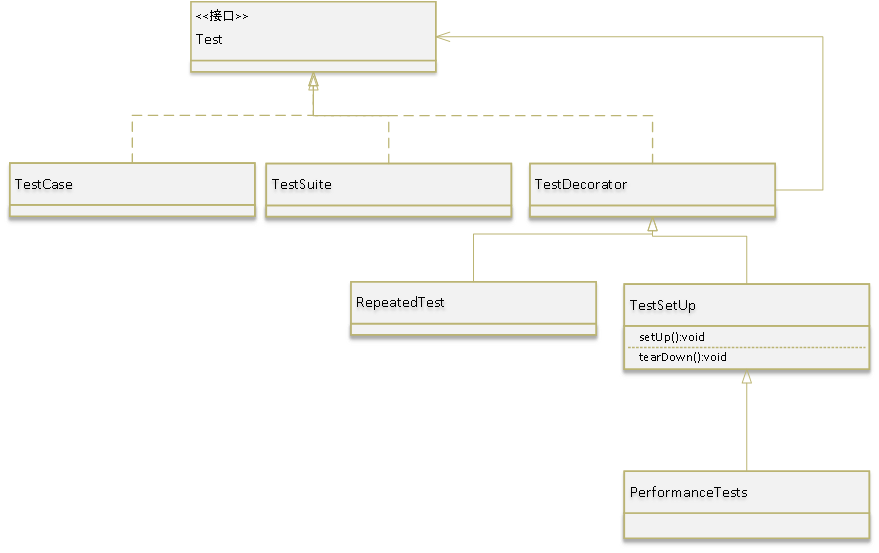
代码示例
public class RepeatedTest extends TestDecorator{
@Override
public void run(TestResult result){
for(int i=0;i<fTimesRepeat;i++){
if(result.shouldStop()){ break; }
super.run(result);
}
}
}
public class TestDecorator extends Assert implements Test{
protected Test fTest;
public TestDecorator(Test test){this.fTest=test;}
public void basicRun(TestResult result){fTest.run(result);}
public int countTestCases(){ return fTest.countTestCases();}
public void run(TestResult result){ basicRun(result);}
public String toString(){return fTest.toString();}
public Test getTest(){ return fTest;}
}
3.1装饰器多次迭代装饰示例:
public interface Anything{
void exe();
}
public class Moon implements Anything{
private Anything a;
public Moon(Anything a){ this.a=a;}
public void exe(){
System.out.println("明月装饰了");
a.exe();
}
}
public class Dream implements Anything{
private Anything a;
public Dream(Anything a){ this.a=a; }
public void exe(){
System.out.println("梦装饰了");
a.exe();
}
}
public class You implements Anything{
private Anything a;
public You(Anything a){ this.a=a; }
public void exe(){
System.out.println("你");
}
}
Anything t=new Moon(new Dream(new You(null)));==>明月装饰了梦装饰了你
Anything t=new Dream(new Moon(new You(null)));==>梦装饰了明月装饰了你
3.2装饰器模式总结:
对象的结构模式
装饰器作用:
不改变对客户端接口的前提下(对客户端透明)
扩展现有对象的功能
思考PerformanceTests的客户端是指谁?
装饰器模式被笼统的成为“包装器”(Wrapper)
适配器也被称为“包装器”,区别在于适配器转换成另一个接口,而装饰器保持接口不变。
包装器形成一条链。
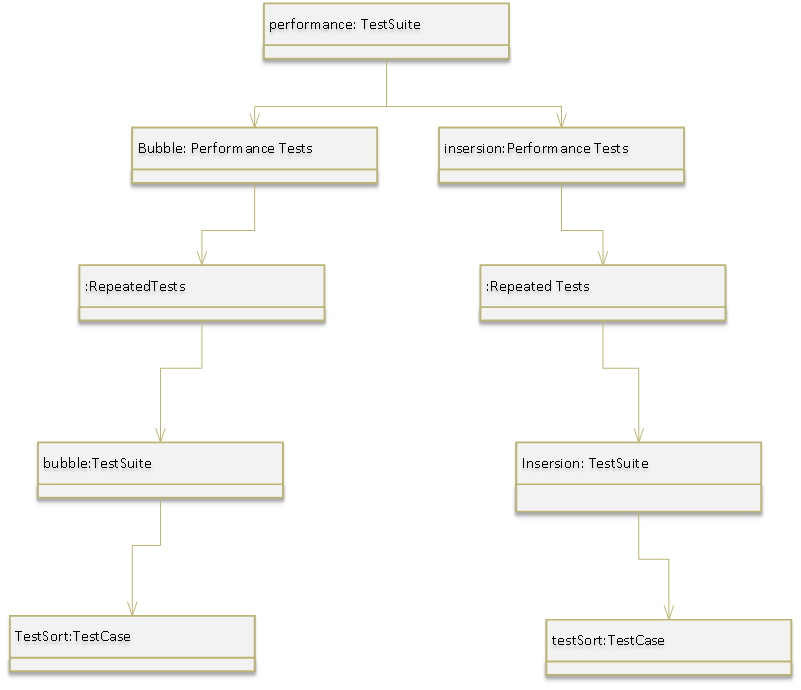
3.3装饰器应用:
Java Servet中的应用:
HttpServletRequest/HttpServletRequestWrapper
HttpServletResponse/HttpServletResponseWrapper
同步化装饰器:
Collections.synchronizedList(list)
取代原先的Vector,HashTable等同步类
Java I/O类库简介:
核心-流,即数据的有序排列,将数据从源送达到目的地
流的种类
--字节流:InputStream,OutputStream
--字符流:Reader,Writer
流的对称性:
--输入- 输出对称
--Byte-Char对称
--学习一种流,可以了解其他所有流。
评论