week3
发布于: 2020 年 10 月 04 日
手写单例
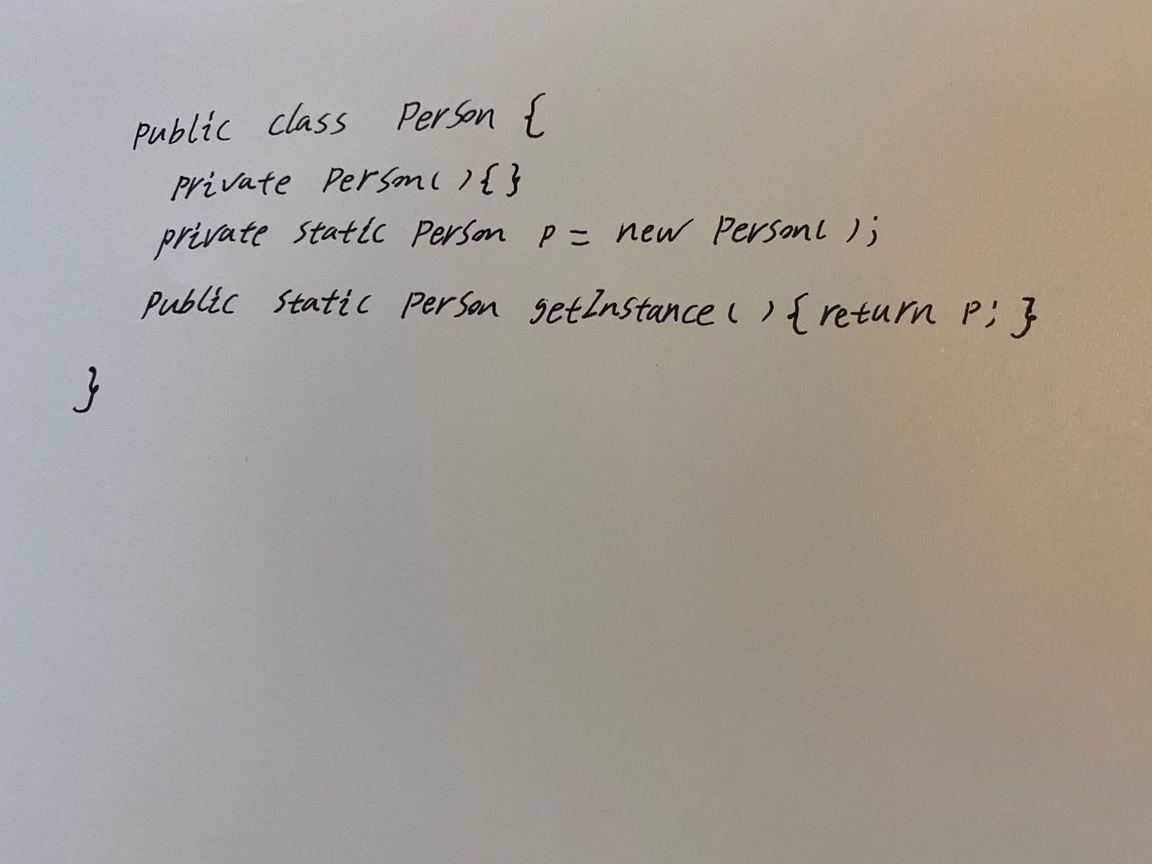
组合模式
a. 定义组件接口
public interface Component { void draw();}
b. 两个抽象类实现接口(基础组件和容器组件)
public abstract class BasicComponent implements Component { private String title; public BasicComponent(String title){ this.title = title; } @Override public void draw(){ drawEle(); }; public String getTitle() { return title; } public abstract void drawEle();}
import java.util.ArrayList;import java.util.List;public abstract class ContainerComponent implements Component { private String title; private List<Component> componentList = new ArrayList<>(); public ContainerComponent(String title){ this.title = title; } @Override public void draw(){ drawEle(); if (componentList.size() > 0) { for (Component component : componentList) { component.draw(); } } } public void addComponent(Component component) { if (component != null) componentList.add(component); } public String getTitle() { return title; } public abstract void drawEle();}
c. 具体的组件继承对应的抽象类,实现drawEle完成自己的输出逻辑
public class WinForm extends ContainerComponent { public WinForm(String title) { super(title); } @Override public void drawEle() { System.out.println(String.format("WinForm(%s)", getTitle())); }}
public class Picture extends BasicComponent { public Picture(String title) { super(title); } @Override public void drawEle() { System.out.println(String.format("Picture(%s)", getTitle())); }}
d. 每个组件都有自己的实现
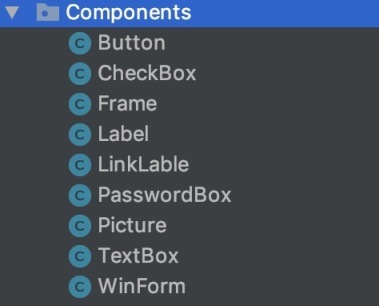
e. 绘制界面
public class Test { public static void main(String[] args) { WinForm win = new WinForm("WINDOW窗口"); win.addComponent(new Picture("LOGO图片")); win.addComponent(new Button("登录")); win.addComponent(new Button("注册")); Frame frame = new Frame("FRAME1"); frame.addComponent(new Label("用户名")); frame.addComponent(new TextBox("文本框")); frame.addComponent(new Label("密码")); frame.addComponent(new PasswordBox("密码框")); frame.addComponent(new CheckBox("复选框")); frame.addComponent(new TextBox("记住用户名")); frame.addComponent(new LinkLable("忘记密码")); win.addComponent(frame); win.draw(); }}
f. 输出结果如下
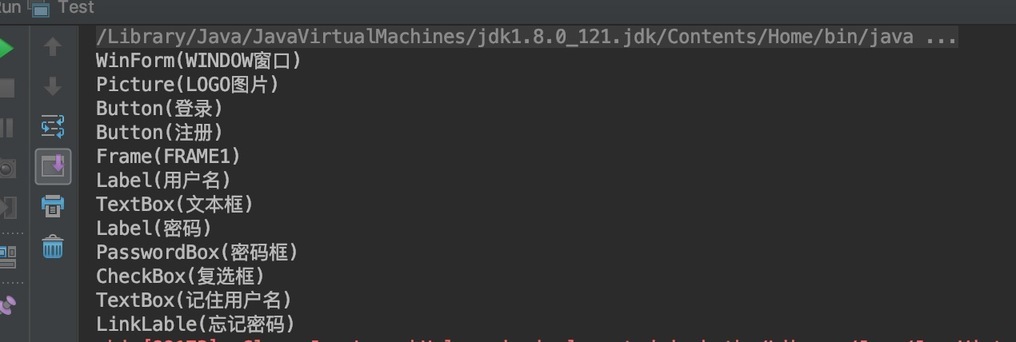
划线
评论
复制
发布于: 2020 年 10 月 04 日 阅读数: 17
张兵
关注
还未添加个人签名 2018.04.25 加入
还未添加个人简介
评论