自动化测试中的 Page Object
发布于: 2021 年 02 月 16 日
前言
最近有人私聊我?
为啥都没看到 python 呢?我只会 python。
好的~
作为一个测试,确实 python 是被比较多测试者接受的语言。(我也许是个例外,2333~)
那么今天我们就用 python 的例子来讲下 页面对象设计模式吧。
一 环境搭建
环境: python 3.6
测试框架:selenium

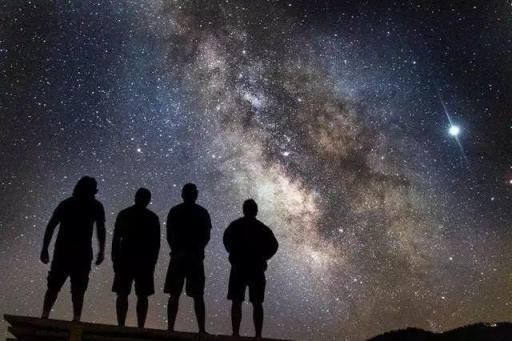

二 简单实例
首先,我们来看下一个最简单的用例。
步骤:打开 google,输入'cheese'
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support.expected_conditions import presence_of_element_located
#This example requires Selenium WebDriver 3.13 or newer
with webdriver.Chrome(executable_path='/*/Downloads/chromedriver') as driver:
wait = WebDriverWait(driver, 10)
driver.get("https://google.com/ncr")
driver.find_element_by_name("q").send_keys("cheese" + Keys.RETURN)
first_result = wait.until(presence_of_element_located((By.CSS_SELECTOR, "h3>div")))
print(first_result.get_attribute("textContent"))
复制代码
在以上的例子中,我们只用了 selenium,接着我们引入 unittest.
import unittest
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
class PythonOrgSearch(unittest.TestCase):
def setUp(self):
path = '/Users/summer.gan/Downloads/chromedriver'
self.driver = webdriver.Chrome(executable_path=path)
def test_search_in_python_org(self):
driver = self.driver
driver.get("http://www.python.org")
self.assertIn("Python", driver.title)
elem = driver.find_element_by_name("q")
elem.send_keys("pycon")
elem.send_keys(Keys.RETURN)
assert "No results found." not in driver.page_source
def tearDown(self):
self.driver.close()
if __name__ == "__main__":
unittest.main()
复制代码
在以上的例子中我们把页面元素,断言,业务流程都写在同个文件中。接下来我们引入 page objects design pattern。
三 page objects design pattern
现在我们来介绍下 page objects design pattern。
使用页面对象设计模式的好处:
创建可跨多个测试用例共用的可重用代码
减少重复代码的数量
如果用户界面发生了变化,修复程序只需要在一个地方进行更改
我们来看下修改后的代码:
testcase.py
import unittest
from selenium import webdriver
import page
class PythonOrgSearch(unittest.TestCase):
"""A sample test class to show how page object works"""
def setUp(self):
path = '/*/Downloads/chromedriver'
self.driver = webdriver.Chrome(executable_path=path)
self.driver.get("http://www.python.org")
def test_search_in_python_org(self):
"""
Tests python.org search feature. Searches for the word "pycon" then verified that some results show up.
Note that it does not look for any particular text in search results page. This test verifies that
the results were not empty.
"""
#Load the main page. In this case the home page of Python.org.
main_page = page.MainPage(self.driver)
#Checks if the word "Python" is in title
assert main_page.is_title_matches(), "python.org title doesn't match."
#Sets the text of search textbox to "pycon"
main_page.search_text_element = "pycon"
main_page.click_go_button()
search_results_page = page.SearchResultsPage(self.driver)
#Verifies that the results page is not empty
assert search_results_page.is_results_found(), "No results found."
def tearDown(self):
self.driver.close()
if __name__ == "__main__":
unittest.main()
复制代码
page.py
from element import BasePageElement
from locators import MainPageLocators
class SearchTextElement(BasePageElement):
"""This class gets the search text from the specified locator"""
#The locator for search box where search string is entered
locator = 'q'
class BasePage(object):
"""Base class to initialize the base page that will be called from all pages"""
def __init__(self, driver):
self.driver = driver
class MainPage(BasePage):
"""Home page action methods come here. I.e. Python.org"""
#Declares a variable that will contain the retrieved text
search_text_element = SearchTextElement()
def is_title_matches(self):
"""Verifies that the hardcoded text "Python" appears in page title"""
return "Python" in self.driver.title
def click_go_button(self):
"""Triggers the search"""
element = self.driver.find_element(*MainPageLocators.GO_BUTTON)
element.click()
class SearchResultsPage(BasePage):
"""Search results page action methods come here"""
def is_results_found(self):
# Probably should search for this text in the specific page
# element, but as for now it works fine
return "No results found." not in self.driver.page_source```
复制代码
element.py
from selenium.webdriver.support.ui import WebDriverWait
class BasePageElement(object):
"""Base page class that is initialized on every page object class."""
def __set__(self, obj, value):
"""Sets the text to the value supplied"""
driver = obj.driver
WebDriverWait(driver, 100).until(
lambda driver: driver.find_element_by_name(self.locator))
driver.find_element_by_name(self.locator).clear()
driver.find_element_by_name(self.locator).send_keys(value)
def __get__(self, obj, owner):
"""Gets the text of the specified object"""
driver = obj.driver
WebDriverWait(driver, 100).until(
lambda driver: driver.find_element_by_name(self.locator))
element = driver.find_element_by_name(self.locator)
return element.get_attribute("value")
复制代码
locator.py
from selenium.webdriver.common.by import By
class MainPageLocators(object):
"""A class for main page locators. All main page locators should come here"""
GO_BUTTON = (By.ID, 'submit')
class SearchResultsPageLocators(object):
"""A class for search results locators. All search results locators should come here"""
pass
复制代码
在 page 类中(page.py),把每个页面创建一个对象,把测试代码与技术实现之间建立一种隔离。
本文主要来自: https://selenium-python.readthedocs.io/page-objects.html
划线
评论
复制
发布于: 2021 年 02 月 16 日阅读数: 12
版权声明: 本文为 InfoQ 作者【夏兮。】的原创文章。
原文链接:【http://xie.infoq.cn/article/1f69831e2d1669fc4de557886】。
本文遵守【CC BY-NC】协议,转载请保留原文出处及本版权声明。
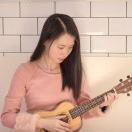
夏兮。
关注
星辰大海... 2018.03.21 加入
测试开发工程师 热爱技术,热爱生活
评论