组合模式 - 树状结构的优雅实现
发布于: 2020 年 06 月 24 日
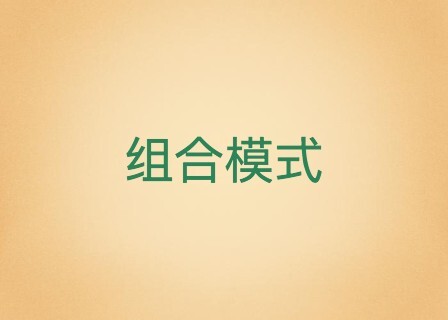
1 二话不说,先上一张手写代码
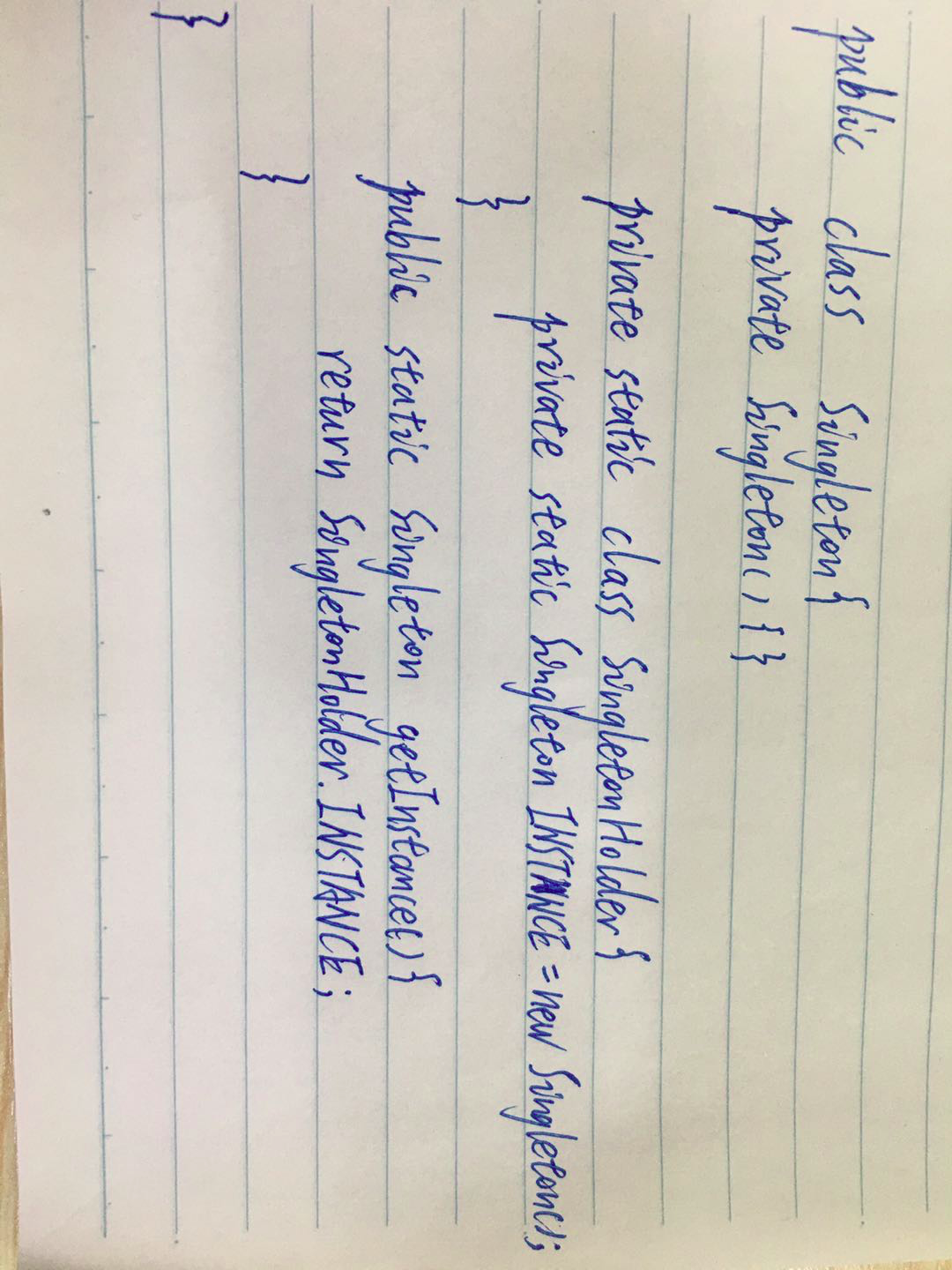
上图为利用内部静态类实现的单例模式,是一种比较优雅的单例模式。
2 树结构实现
开始进入我们的正题,在编程实践中,经常会遇到树状结构的场景,比如我们的浏览器窗口,比如文件系统。
那么,在处理树状结构的时候有什么较好的方式呢?
现在,我们就来学习一种利用组合模式的方法。
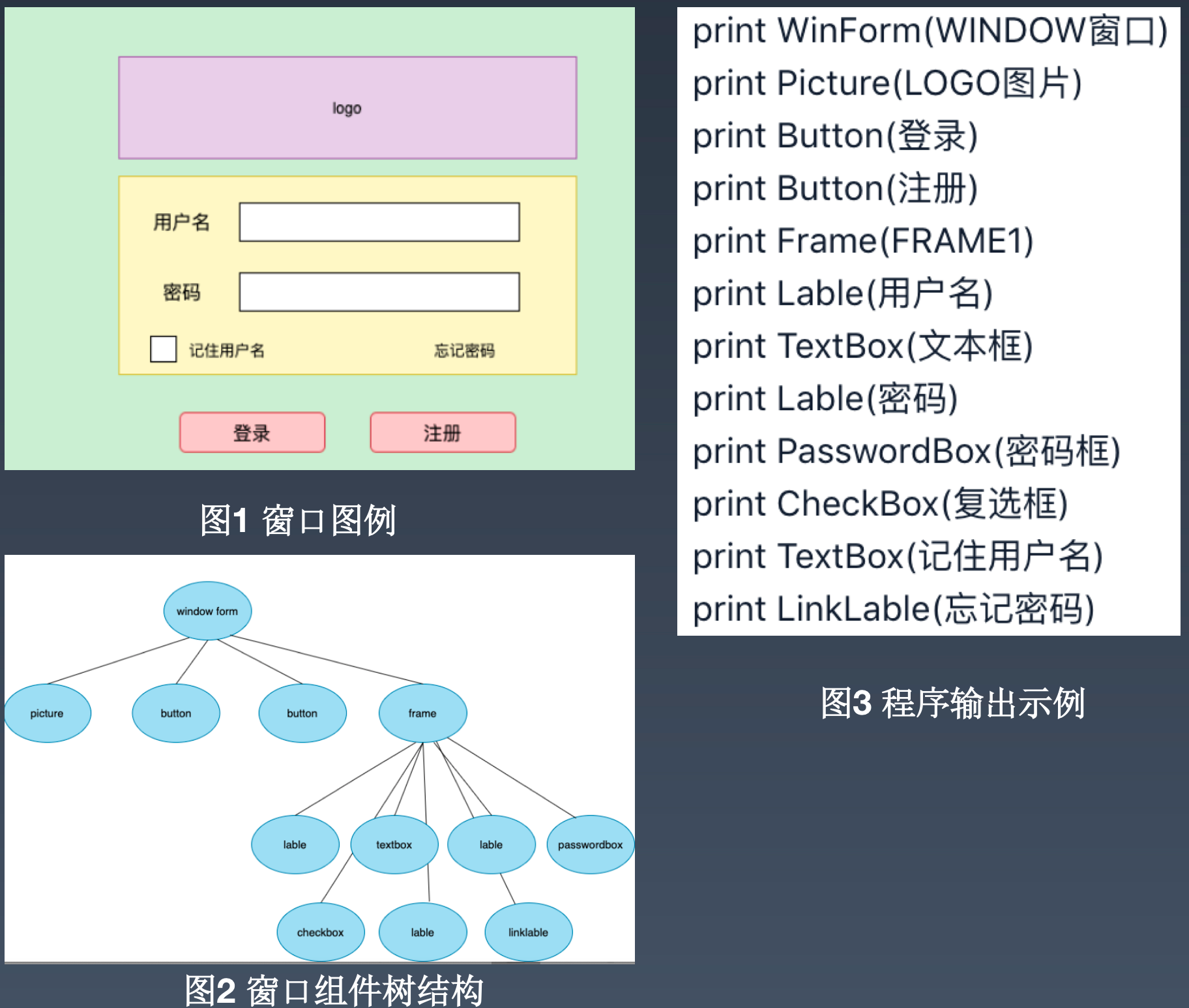
如上图所示,我们通过程序输出的方式模拟窗口渲染的过程。
下面直接上代码,首先定义一个接口,所有的组件都需要实现这个接口。
public interface ViewTree { void draw();}
定义窗口类:
public class TreeWindow implements ViewTree { private String windowName; private List<ViewTree> subTree; public TreeWindow(String windowName) { this.subTree = new ArrayList<>(); this.windowName = windowName; } @Override public void draw() { System.out.println("开始渲染:WinForm("+windowName+")"); for (ViewTree viewTree : subTree){ viewTree.draw(); } } public void addSub(ViewTree viewTree){ subTree.add(viewTree); }}
定义图片组件类:
public class TreePicture implements ViewTree { private String pictureName; public TreePicture(String pictureName) { this.pictureName = pictureName; } @Override public void draw() { System.out.println("开始渲染:Picture("+pictureName+")"); }}
定义Botton组件类:
public class TreeButton implements ViewTree { private String buttonName; public TreeButton(String buttionName) { this.buttonName = buttionName; } @Override public void draw() { System.out.println("开始渲染:Buttion("+ buttonName +")"); }}
定义Frame组件类:
public class TreeFrame implements ViewTree { private String frameName; private List<ViewTree> subTree; public TreeFrame(String frameName) { this.frameName = frameName; this.subTree = new ArrayList<>(); } @Override public void draw() { System.out.println("开始渲染:Frame("+ frameName +")"); for (ViewTree viewTree : subTree){ viewTree.draw(); } } public void addSub(ViewTree viewTree){ subTree.add(viewTree); }}
定义Lable组件类:
public class TreeLable implements ViewTree { private String lableName; public TreeLable(String lableName) { this.lableName = lableName; } @Override public void draw() { System.out.println("开始渲染:Lable("+ lableName +")"); }}
定义TextBox组件类:
public class TreeTextBox implements ViewTree { private String textBoxName; public TreeTextBox(String textBoxName) { this.textBoxName = textBoxName; } @Override public void draw() { System.out.println("开始渲染:TextBox("+ textBoxName +")"); }}
定义PasswordBox组件类:
public class TreePasswordBox implements ViewTree { private String passwordBoxName; public TreePasswordBox(String passwordBoxName) { this.passwordBoxName = passwordBoxName; } @Override public void draw() { System.out.println("开始渲染:PasswordBox("+ passwordBoxName +")"); }}
定义CheckBox组件类:
public class TreeCheckBox implements ViewTree { private String checkBoxName; public TreeCheckBox(String checkBoxName) { this.checkBoxName = checkBoxName; } @Override public void draw() { System.out.println("开始渲染:CheckBox("+ checkBoxName +")"); }}
定义LinkLable组件类:
public class TreeLinkLable implements ViewTree { private String linkLableName; public TreeLinkLable(String linkLableName) { this.linkLableName = linkLableName; } @Override public void draw() { System.out.println("开始渲染:LinkLable("+ linkLableName +")"); }}
最后定义Main类,组装窗口:
public class Main { public static void main(String[] args){ //初始化窗口 TreeWindow window = new TreeWindow("WINDOW窗口"); //window添加组件 TreePicture picture = new TreePicture("LOGO图片"); TreeButton login = new TreeButton("登录"); TreeButton register = new TreeButton("注册"); TreeFrame frame = new TreeFrame("FRAME1"); window.addSub(picture); window.addSub(login); window.addSub(register); window.addSub(frame); //frame添加组件 TreeLable userNameLable = new TreeLable("用户名"); TreeTextBox userNameBox = new TreeTextBox("文本框"); TreeLable passwordLable = new TreeLable("密码"); TreePasswordBox passwordBox = new TreePasswordBox("密码框"); TreeCheckBox checkBox = new TreeCheckBox("复选框"); TreeTextBox rememberUserNameBox = new TreeTextBox("记住用户名"); TreeLinkLable linkLable = new TreeLinkLable("忘记密码"); frame.addSub(userNameLable); frame.addSub(userNameBox); frame.addSub(passwordLable); frame.addSub(passwordBox); frame.addSub(checkBox); frame.addSub(rememberUserNameBox); frame.addSub(linkLable); //开始渲染 window.draw(); }}
控制台输入:
开始渲染:WinForm(WINDOW窗口)开始渲染:Picture(LOGO图片)开始渲染:Buttion(登录)开始渲染:Buttion(注册)开始渲染:Frame(FRAME1)开始渲染:Lable(用户名)开始渲染:TextBox(文本框)开始渲染:Lable(密码)开始渲染:PasswordBox(密码框)开始渲染:CheckBox(复选框)开始渲染:TextBox(记住用户名)开始渲染:LinkLable(忘记密码)
好了,关于利用组合模式优雅的实现树状结构的全部内容就到这里结束了,感谢查阅。
划线
评论
复制
发布于: 2020 年 06 月 24 日 阅读数: 31
版权声明: 本文为 InfoQ 作者【hellohuan】的原创文章。
原文链接:【http://xie.infoq.cn/article/1de704d02dec929cfbaa2c784】。文章转载请联系作者。
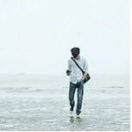
hellohuan
关注
活到老,学到老 2018.09.17 加入
从事互联网研发工作,对产品、运营充满兴趣,终身学习践行者
评论