架構師訓練營第 1 期 - 第 03 周作業
发布于: 2020 年 10 月 04 日
1. 请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
class Singleton {
public:
static Singleton& getInstance() {
static Singleton instance;
return instance;
}
void show(){
std::cout << "Singleton\n";
}
private:
Singleton() = default;
~Singleton() = default;
Singleton(const Singleton& instance) = delete;
Singleton& operator=(const Singleton& instance) = delete;
};
复制代码
2. 请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
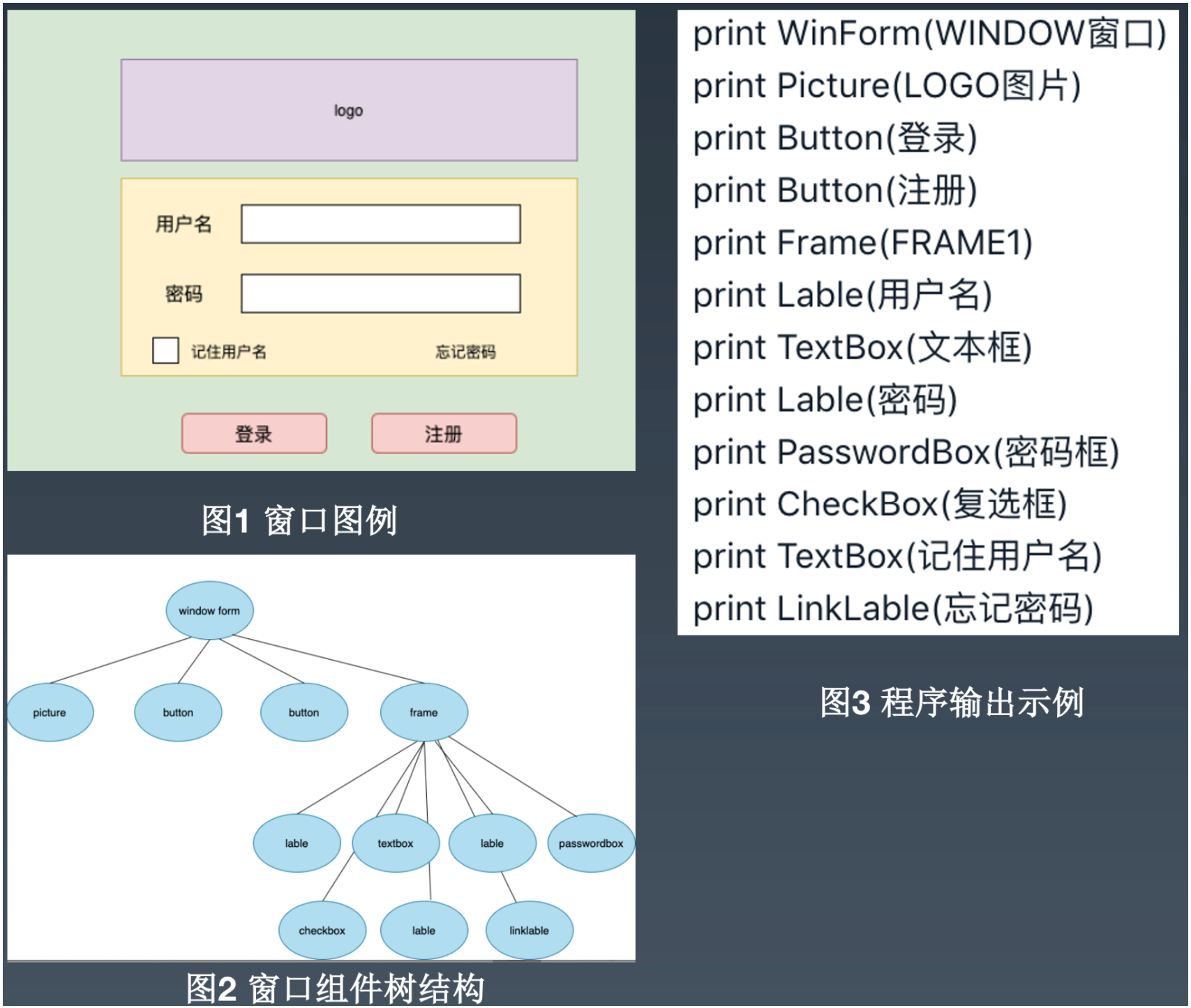
圖 2 窗口組件數結構與圖 3 程序輸出示例中,"記住用戶名"的部分不一致
圖 2 為 Label
圖 3 卻顯示為 TextBox
參考圖 1 的圖例,決定採用圖 2 的 Label 組件
#include <iostream>
#include <vector>
class Component {
public:
Component(const std::string& name): _name(name){};
virtual void draw() = 0;
virtual ~Component() = default;
protected:
std::string _name;
};
class Container : public Component {
public:
Container(const std::string& name): Component(name){};
void addComponent(Component& com) {_comList.push_back(&com);};
virtual void draw();
~Container() = default;
private:
std::vector<Component*> _comList;
};
void Container::draw() {
for (auto& item : _comList) {
item->draw();
}
}
class WinForm : public Container{
public:
WinForm(const std::string name): Container(name){};
virtual void draw();
~WinForm() = default;
};
void WinForm::draw() {
std::cout << "WinForm (" << _name << ")\n";
Container::draw();
}
class Frame : public Container{
public:
Frame(const std::string name): Container(name){};
virtual void draw();
~Frame() = default;
};
void Frame::draw() {
std::cout << "Frame (" << _name << ")\n";
Container::draw();
}
class Picture : public Component {
public:
Picture(const std::string& name) : Component(name){};
virtual void draw() {std::cout << "Picture (" << _name << ")\n";};
~Picture() = default;
};
class Button : public Component {
public:
Button(const std::string& name) : Component(name){};
virtual void draw() {std::cout << "Button (" << _name << ")\n";};
~Button() = default;
};
class Label : public Component {
public:
Label(const std::string& name) : Component(name){};
virtual void draw() {std::cout << "Label (" << _name << ")\n";};
~Label() = default;
};
class LinkLabel : public Component {
public:
LinkLabel(const std::string& name) : Component(name){};
virtual void draw() {std::cout << "LinkLabel (" << _name << ")\n";};
~LinkLabel() = default;
};
class TextBox : public Component {
public:
TextBox(const std::string& name) : Component(name){};
virtual void draw() {std::cout << "TextBox (" << _name << ")\n";};
~TextBox() = default;
};
class PasswordBox : public Component {
public:
PasswordBox(const std::string& name) : Component(name){};
virtual void draw() {std::cout << "PasswordBox (" << _name << ")\n";};
~PasswordBox() = default;
};
class CheckBox : public Component {
public:
CheckBox(const std::string& name) : Component(name){};
virtual void draw() {std::cout << "CheckBox (" << _name << ")\n";};
~CheckBox() = default;
};
int main(){
WinForm win = WinForm("Window窗口");
Picture pic = Picture("Logo圖片");
Button btn1 = Button("登錄");
Button btn2 = Button("註冊");
Frame frame = Frame("Frame1");
Label label1 = Label("用戶名");
TextBox textB1 = TextBox("文本框");
Label label2 = Label("密碼");
PasswordBox passB = PasswordBox("密碼框");
CheckBox checkB = CheckBox("複選框");
Label label3 = Label("記住用戶名");
LinkLabel link = LinkLabel("忘記密碼");
win.addComponent(pic);
win.addComponent(btn1);
win.addComponent(btn2);
win.addComponent(frame);
frame.addComponent(label1);
frame.addComponent(textB1);
frame.addComponent(label2);
frame.addComponent(passB);
frame.addComponent(checkB);
frame.addComponent(label3);
frame.addComponent(link);
win.draw();
}
复制代码
划线
评论
复制
发布于: 2020 年 10 月 04 日阅读数: 37
版权声明: 本文为 InfoQ 作者【Panda】的原创文章。
原文链接:【http://xie.infoq.cn/article/1c50ae5a47b43915723872860】。未经作者许可,禁止转载。
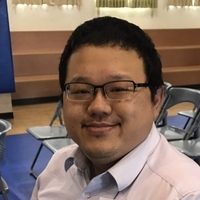
Panda
关注
还未添加个人签名 2015.06.29 加入
还未添加个人简介
评论