小结 3
Singleton pattern
Singleton pattern is one of the simplest design patterns in Java. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object.
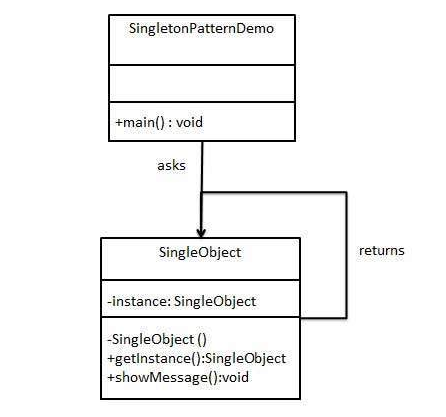
Builder pattern builds a complex object using simple objects and using a step by step approach. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object.
Decorator pattern allows a user to add new functionality to an existing object without altering its structure. This type of design pattern comes under structural pattern as this pattern acts as a wrapper to existing class.
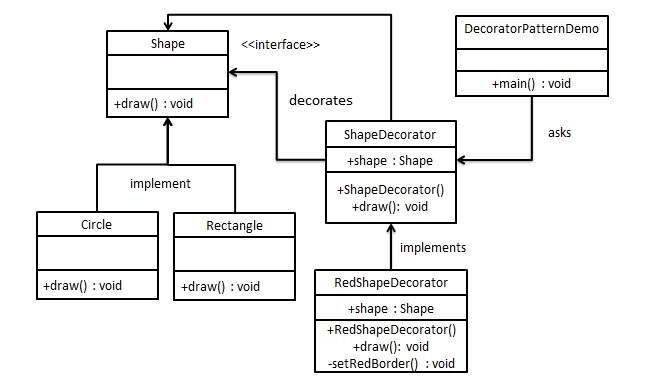
小结:
重新把Design Patterns查了一遍。发现有很多的模式都淡忘了。接下来,需要把所以的patterns都过一遍。(Builder Pattern, Prototype Pattern, Adapter Pattern, Bridge Pattern, Decorator Pattern, Proxy Pattern.....)
refer to:
https://www.tutorialspoint.com/design_pattern/builder_pattern.htm
评论