组合模式
发布于: 2020 年 11 月 08 日
golang 设计模式
https://github.com/kaysun/go_design_pattern.git
题目:
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
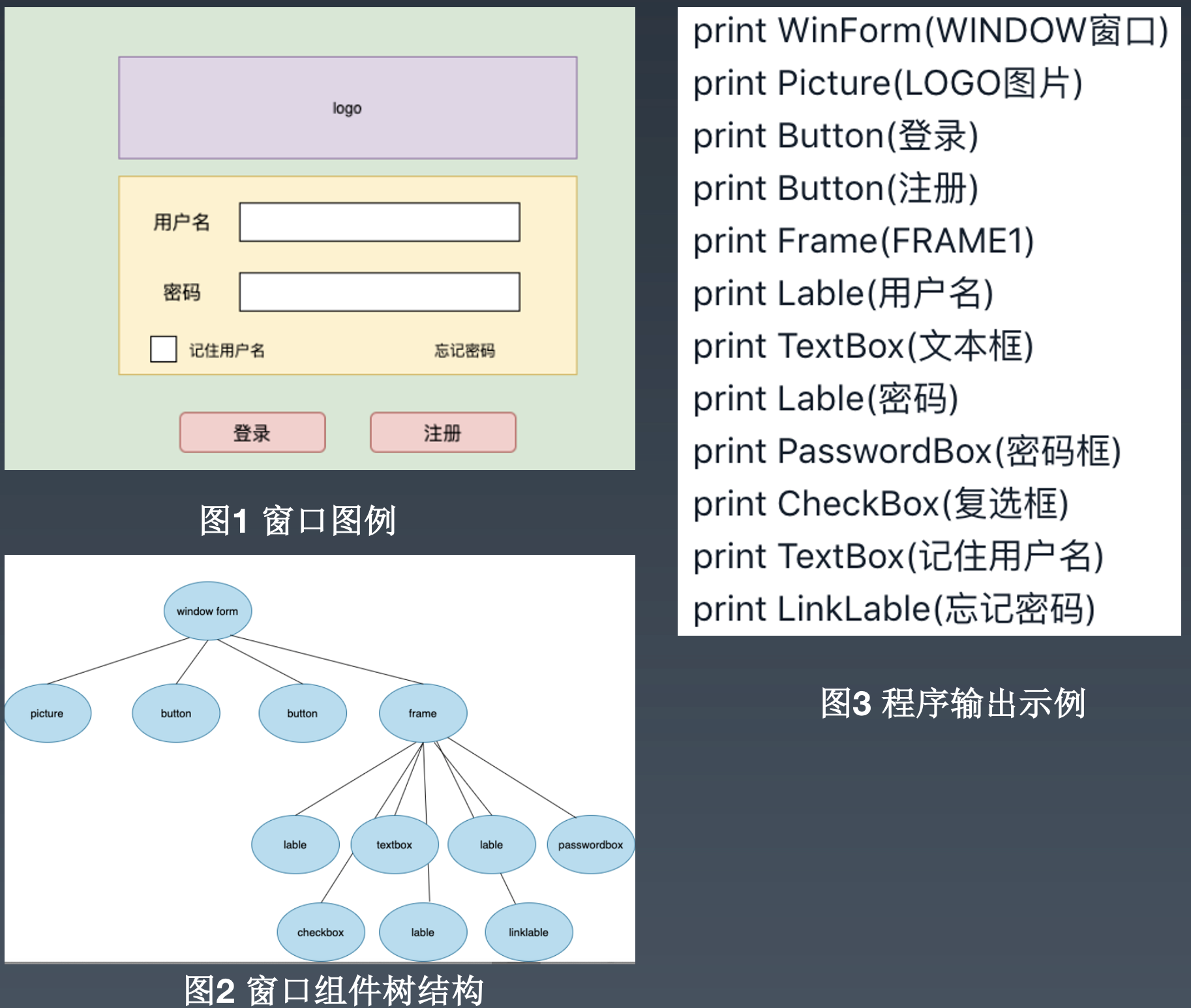
component.go
//package combination 组合模式
package combination
// UIComponent UI组件接口,对于任何UI控件都适用。
type UIComponent interface {
// PrintUIComponent 打印UI组件
PrintUIComponent()
// GetUIControlName 获取控件名字
GetUIControlName() string
// GetConcreteUIControlName 获取控件具体名字
GetConcreteUIControlName() string
}
// UIComponentAddtion UI组件附加接口,使用接口隔离原则,保证不需要实现接口声明方法的结构体,没有额外负担。仅对容器类型对UI控件适用。
type UIComponentAddtion interface {
// AddUIComponent 添加UI组件
AddUIComponent(component UIComponent)
// AddUIComponents 添加UI组件列表
AddUIComponents(components []UIComponent)
// GetUIComponentList 获取UI组件列表
GetUIComponentList() []UIComponent
}
// UIAttr UI属性
type UIAttr struct {
// UI 名字
Name string
}
//client 打印client
var client = &PrintClient{}
复制代码
container.go
package combination
// WinForm 窗口,实现UIComponent、UIComponentAddtion接口
type WinForm struct {
// UIAttr 嵌套UI属性
UIAttr
// Components 容器的组件列表
Components []UIComponent
}
// GetUIControlName 获取控件名字
func (window *WinForm) GetUIControlName() string {
return "WinForm"
}
// GetConcreteUIControlName 获取控件具体名字
func (window *WinForm) GetConcreteUIControlName() string {
return window.Name
}
// PrintUIComponent 打印UI组件
func (window *WinForm) PrintUIComponent() {
client.printContainer(window, window)
}
// AddUIComponent 添加UI组件
func (window *WinForm) AddUIComponent(component UIComponent) {
window.Components = append(window.Components, component)
}
// AddUIComponents 添加UI组件列表
func (window *WinForm) AddUIComponents(components []UIComponent) {
window.Components = append(window.Components, components...)
}
// GetUIComponentList 获取UI组件列表
func (window *WinForm) GetUIComponentList() []UIComponent {
return window.Components
}
// Frame 框架,实现UIComponent、接口
type Frame struct {
// UIAttr 嵌套UI属性
UIAttr
// Components 容器的组件列表
Components []UIComponent
}
// GetUIControlName 获取控件名字
func (frame *Frame) GetUIControlName() string {
return "Frame"
}
// GetConcreteUIControlName 获取控件具体名字
func (frame *Frame) GetConcreteUIControlName() string {
return frame.Name
}
// PrintUIComponent 打印UI组件
func (frame *Frame) PrintUIComponent() {
client.printContainer(frame, frame)
}
// AddUIComponent 添加UI组件
func (frame *Frame) AddUIComponent(component UIComponent) {
frame.Components = append(frame.Components, component)
}
// AddUIComponents 添加UI组件列表
func (frame *Frame) AddUIComponents(components []UIComponent) {
frame.Components = append(frame.Components, components...)
}
// GetUIComponentList 获取UI组件列表
func (frame *Frame) GetUIComponentList() []UIComponent {
return frame.Components
}
复制代码
leaf.go
package combination
// Picture 图片,实现UIComponent接口
type Picture struct {
// UIAttr 嵌套UI属性
UIAttr
}
// GetUIControlName 获取控件名字
func (picture Picture) GetUIControlName() string {
return "Picture"
}
// GetConcreteUIControlName 获取控件具体名字
func (picture Picture) GetConcreteUIControlName() string {
return picture.Name
}
// PrintUIComponent 打印UI组件
func (picture Picture) PrintUIComponent() {
client.printCurrentControl(picture)
}
// Button 按钮,实现UIComponent接口
type Button struct {
// UIAttr 嵌套UI属性
UIAttr
}
// GetUIControlName 获取控件名字
func (button Button) GetUIControlName() string {
return "Button"
}
// GetConcreteUIControlName 获取控件具体名字
func (button Button) GetConcreteUIControlName() string {
return button.Name
}
// PrintUIComponent 打印UI组件
func (button Button) PrintUIComponent() {
client.printCurrentControl(button)
}
// Label 标签,实现UIComponent接口
type Label struct {
// UIAttr 嵌套UI属性
UIAttr
}
// GetUIControlName 获取控件名字
func (label Label) GetUIControlName() string {
return "Label"
}
// GetConcreteUIControlName 获取控件具体名字
func (label Label) GetConcreteUIControlName() string {
return label.Name
}
// PrintUIComponent 打印UI组件
func (label Label) PrintUIComponent() {
client.printCurrentControl(label)
}
// TextBox 文本框,实现UIComponent接口
type TextBox struct {
// UIAttr 嵌套UI属性
UIAttr
}
// GetUIControlName 获取控件名字
func (textBox TextBox) GetUIControlName() string {
return "TextBox"
}
// GetConcreteUIControlName 获取控件具体名字
func (textBox TextBox) GetConcreteUIControlName() string {
return textBox.Name
}
// PrintUIComponent 打印UI组件
func (textBox TextBox) PrintUIComponent() {
client.printCurrentControl(textBox)
}
// PassWordBox 密码框,实现UIComponent接口
type PassWordBox struct {
// UIAttr 嵌套UI属性
UIAttr
}
// GetUIControlName 获取控件名字
func (passWordBox PassWordBox) GetUIControlName() string {
return "PassWordBox"
}
// GetConcreteUIControlName 获取控件具体名字
func (passWordBox PassWordBox) GetConcreteUIControlName() string {
return passWordBox.Name
}
// PrintUIComponent 打印UI组件
func (passWordBox PassWordBox) PrintUIComponent() {
client.printCurrentControl(passWordBox)
}
// CheckBox 复选框,实现UIComponent接口
type CheckBox struct {
// UIAttr 嵌套UI属性
UIAttr
}
// GetUIControlName 获取控件名字
func (checkBox CheckBox) GetUIControlName() string {
return "CheckBox"
}
// GetConcreteUIControlName 获取控件具体名字
func (checkBox CheckBox) GetConcreteUIControlName() string {
return checkBox.Name
}
// PrintUIComponent 打印UI组件
func (checkBox CheckBox) PrintUIComponent() {
client.printCurrentControl(checkBox)
}
// LinkLabel 关联的标签,实现UIComponent接口
type LinkLabel struct {
// UIAttr 嵌套UI属性
UIAttr
}
// GetUIControlName 获取控件名字
func (linkLabel LinkLabel) GetUIControlName() string {
return "LinkLabel"
}
// GetConcreteUIControlName 获取控件具体名字
func (linkLabel LinkLabel) GetConcreteUIControlName() string {
return linkLabel.Name
}
// PrintUIComponent 打印UI组件
func (linkLabel LinkLabel) PrintUIComponent() {
client.printCurrentControl(linkLabel)
}
复制代码
client.go
package combination
import "fmt"
// PrintClient 打印客户端
type PrintClient struct{}
// printContainer 打印容器控件
func (client PrintClient) printContainer(component UIComponent, componentAddtion UIComponentAddtion) {
client.printCurrentControl(component)
for _, v := range componentAddtion.GetUIComponentList() {
v.PrintUIComponent()
}
}
// printCurrentControl 打印当前控件
func (client PrintClient) printCurrentControl(component UIComponent) {
fmt.Println(fmt.Sprintf("print %s(%s)", component.GetUIControlName(), component.GetConcreteUIControlName()))
}
复制代码
component_test.go
package combination
import (
"testing"
)
func Test(t *testing.T) {
t.Run("combination: ", Combination)
}
// Combination 单元测试
//print WinForm(WINDOW窗口)
//print Picture(LOGO图片)
//print Button(登录)
//print Button(注册)
//print Frame(FRAME1)
//print Label(用户名)
//print TextBox(文本框)
//print Label(密码)
//print PassWordBox(密码框)
//print CheckBox(复选框)
//print TextBox(记住用户名)
//print LinkLabel(忘记密码)
func Combination(t *testing.T) {
window := &WinForm{UIAttr: UIAttr{Name: "WINDOW窗口"}}
picture := &Picture{UIAttr{Name: "LOGO图片"}}
loginButton := &Button{UIAttr{Name: "登录"}}
registerButton := &Button{UIAttr{Name: "注册"}}
frame := &Frame{UIAttr: UIAttr{Name: "FRAME1"}}
userLable := &Label{UIAttr{Name: "用户名"}}
textBox := &TextBox{UIAttr{Name: "文本框"}}
passwordLable := &Label{UIAttr{Name: "密码"}}
passwordBox := &PassWordBox{UIAttr{Name: "密码框"}}
checkBox := &CheckBox{UIAttr{Name: "复选框"}}
rememberUserTextBox := &TextBox{UIAttr{Name: "记住用户名"}}
linkLable := &LinkLabel{UIAttr{Name: "忘记密码"}}
window.AddUIComponents([]UIComponent{picture, loginButton, registerButton, frame})
frame.AddUIComponents([]UIComponent{userLable, textBox, passwordLable, passwordBox, checkBox, rememberUserTextBox, linkLable})
window.PrintUIComponent()
}
复制代码
划线
评论
复制
发布于: 2020 年 11 月 08 日阅读数: 46
版权声明: 本文为 InfoQ 作者【猴子胖胖】的原创文章。
原文链接:【http://xie.infoq.cn/article/0fa2a3d3861b4b9b5c47eadf2】。文章转载请联系作者。
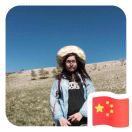
猴子胖胖
关注
6年ios开发,1年golang开发 2020.05.09 加入
还未添加个人简介
评论