ARTS 第 5 周
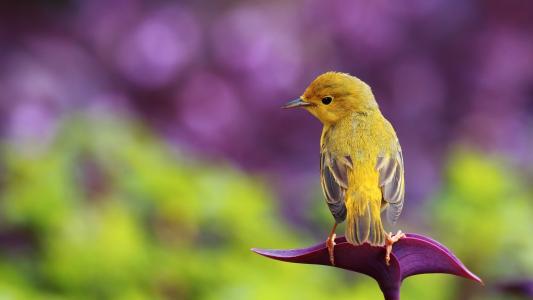
每周完成一个 ARTS:
Algorithm: 每周至少做一个 LeetCode 的算法题
Review: 阅读并点评至少一篇英文技术文章
Tips: 学习至少一个技术技巧
Share: 分享一篇有观点和思考的技术文章(一周一篇强度有点大,视情况而定)
Algorithm
本周做了112. 路径总和,我使用的深度遍历是实现,用广度遍历空间复杂度会低一点。
还有一种广度遍历的解法,
BFS
Review
本周阅读的是《Spring Cloud 官方文档》,因为最近在学这个,硬着头皮配合谷歌读的。
综述
Spring Cloud为开发人员提供了工具来快速构建分布式系统中的某些常见模式(例如配置管理,服务发现,断路器,智能路由,微代理,控制总线)。
Distributed/versioned configuration 分布式/版本化配置
Service registration and discovery 服务注册与发现
Routing 路由
Service-to-service calls 服务间呼叫
Load balancing 负载均衡
Circuit Breakers 熔断器
Distributed messaging 分布式消息传递
Tip
分布式锁,使用注解实现。
这是一种面向切面的设计模式,被注解的方法在运行前会去获取redis锁,运行结束后释放锁。
主要靠aspectj类实现切面。
注解类
切面类
share
本周无分享
评论