ARTS - Week Five
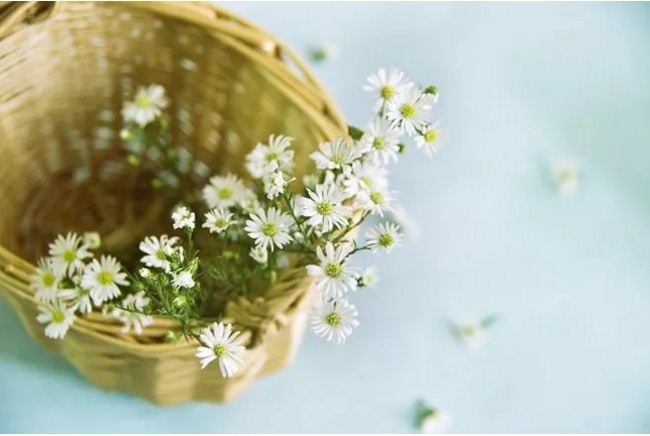
Algorithm
Problem
42. Trapping Rain Water
Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it is able to trap after raining.
Solution
Review
Artical
Vue 2 props and emit - parent to child
Link
https://medium.com/@sky790312/about-vue-2-ops-af3b5bb59829
Review
The correct way to use props and $emit
OK, so what’s correct way ? we want component reusable, it should pure. Following is the flow what vue design.
Parent has data to control, pass data to child component by props.
If child component need to deal with props data, assign props data value to itself’s data when component mounted.
After handle method, parent open a interface to child. Child $emit back new value to parent.
(every component has it scope and data.)
Tips
Dynamic directive arguments
One of the coolest features of Vue 2.6 is the possibility to pass down directive arguments to a component dynamically. Imagine you have a Button-Component and want to listen to a Click-Event in some cases but to a DoubleClick-Event in other cases. That's where those directives come in handy:
Share
Artical
7 Simple VueJS Tips You Can Use to Become a Better Developer
Link
https://medium.com/swlh/7-simple-vuejs-tips-you-can-use-to-become-a-better-developer-dd423db07881
Summary
How to write optimized JavaScript
1. Use one component in multiple routes
2. The $on(‘hook:’) can help simplify your code
3. $on can also listen to a child component’s lifecycle hooks
4. Use immediate: true to trigger watchers on initialization
5. You should always validate your props…please.
6. Passing all props to a child component is easy
7. You HAVE TO understand all the component options
when would I use a watcher?
Observing a value until it reaches a specific value
Making an asynchronous API call when a value changes
You don’t need to combine existing data into a new property
VueJS Lifecycle Hooks
Using the beforeCreate hook is useful when you need some sort of logic/API call that does not need to be assigned to data.
Mounting Hooks – Accessing the DOM
Update Hooks – Reactivity in the VueJS Lifecycle
Destruction Hooks – Cleaning Things Up
How to Manage VueJS Mixins
评论