ARTS - Week One
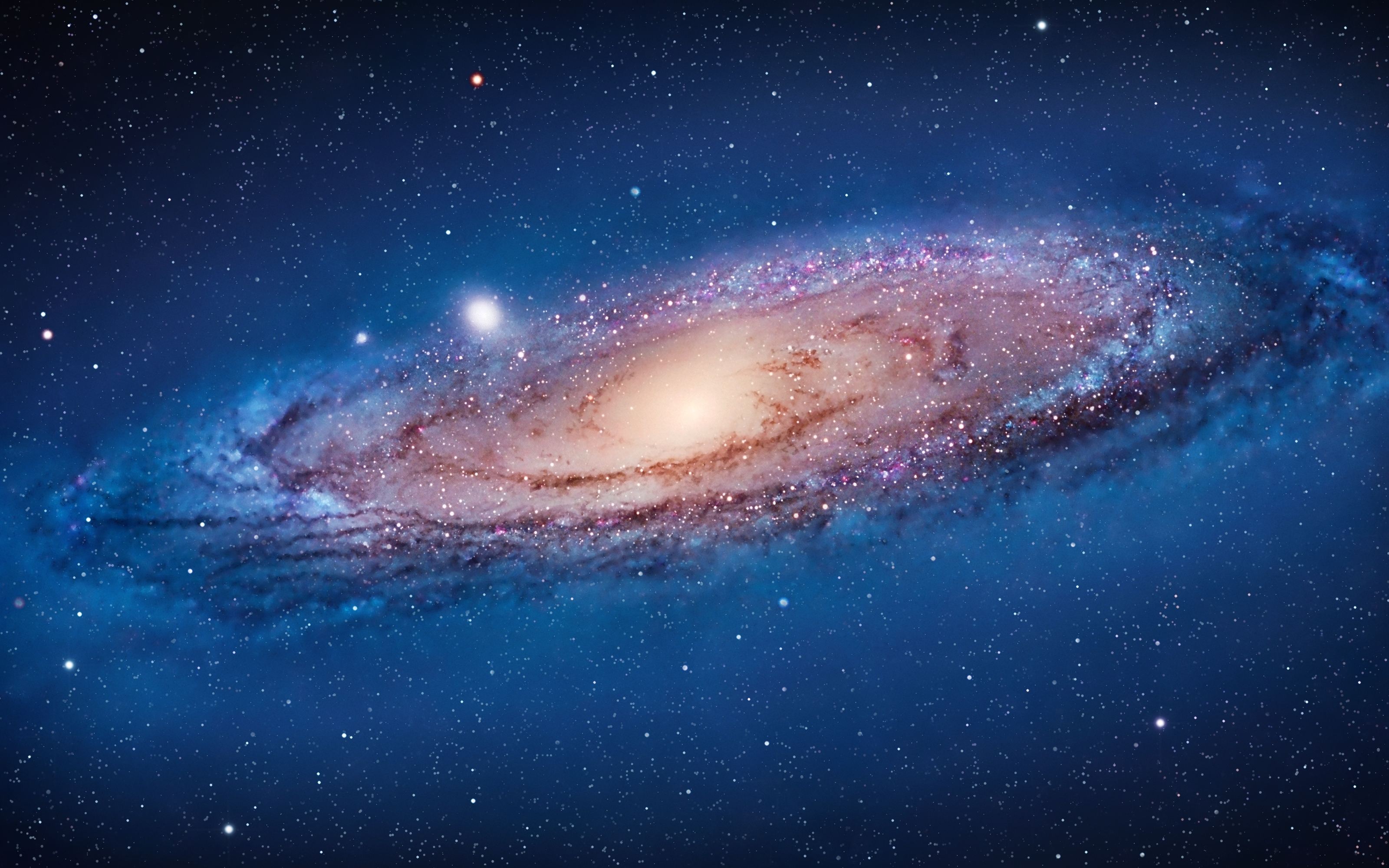
Algorithm
Problem
Reverse Integer - From LeetCode
Given a 32-bit signed integer, reverse digits of an integer.
Solution
Thinking
It's just a beginning, and making a small attempt.
Review
Artical
The Key To Accelerating Your Coding Skills
Link
http://blog.thefirehoseproject.com/posts/learn-to-code-and-be-self-reliant/
Review
The final stage of the inflection point process is acceptance. Acceptance that software development is a process of continuous learning. Acceptance that the feeling that you’ve successfully learned everything just means you should start thinking about solving more complicated problems.
As a programmer,I just accept the happy fact, work and learn, live and learn. Keep learning!
Tips
Programs often waste time calling functions which recalculate the same results over and over again.We can use memorization to solve this problem by caching its previously computed results.
For example:
Share
Artical
Glossary of Modern JavaScript Concepts: Part 1
Link
https://auth0.com/blog/glossary-of-modern-javascript-concepts/
Summary
1.Purity: Pure Functions, Impure Functions, Side Effects
Pure functions must take arguments.
The same input (arguments) will always produce the same output (return).
Pure functions rely only on local state and do not mutate external state (note:
console.log
changes global state).Pure functions do not produce side effects.
Pure functions cannot call impure functions.
Impure function mutates state outside its scope. Any function that has side effects (see below) is impure.
When a function or expression modifies state outside its own context, the result is a side effect. Include making a call to an API, manipulating the DOM, raising an alert dialog, writing to a database, etc.
2.State
State refers to the information a program has access to and can operate on at a point in time.
Stateful programs, apps, or components store data in memory about the current state.
Stateless functions or components perform tasks as though running them for the first time, every time. Pure functions are stateless.
3.Immutability and Mutability
If an object is immutable, its value cannot be modified after creation.
If an object is mutable, its value can be modified after creation.
Immutability's advantage: It results in code that is simpler to reason about; It also enables persistency.
Immutability's disadvantage: many algorithms and operations cannot be implemented efficiently.
4.Imperative and Declarative Programming
Imperative programming describes how a program's logic works in explicit commands with statements that modify the program state.
Declarative programming describes what a program's logic accomplishes without describing how.
5.Higher-order Functions
accepts another function as an argument, or returns a function as a result.
6.Functional Programming
Core functionality is implemented using pure functions without side effects.
Data is immutable.
Functional programs are stateless.
Imperative container code manages side effects and executes declarative, pure core code.
7.Observables
User interface events like button clicks, mouse movement, etc. are hot. Hot observables will always push even if we're not specifically reacting to them with a subscription.
A cold observable begins pushing only when we subscribe to it. If we subscribe again, it will start over.
8.Reactive Programming
Reactive programming is concerned with propagating and responding to incoming events over time, declaratively (describing what to do rather than how).
Reactive programming is often associated with Reactive Extensions.
9.Functional Reactive Programming
denotative: the meaning of each function or type is precise, simple, and implementation-independent ("functional" references this)
continuous time: variables have a particular value for a very short time: between any two points are an infinite number of other points; provides transformation flexibility, efficiency, modularity, and accuracy ("reactive" references this)
Functional reactive programming should be:
dynamic: can react over time or to input changes
time-varying: reactive behaviors can change continually while reactive values change discretely
efficient: minimize amount of processing necessary when inputs change
historically aware: pure functions map state from a previous point in time to the next point in time; state changes concern the local element and not the global program state
评论 (1 条评论)