架构 1 期第三周作业一
发布于: 2020 年 09 月 28 日
1、手写单例模式
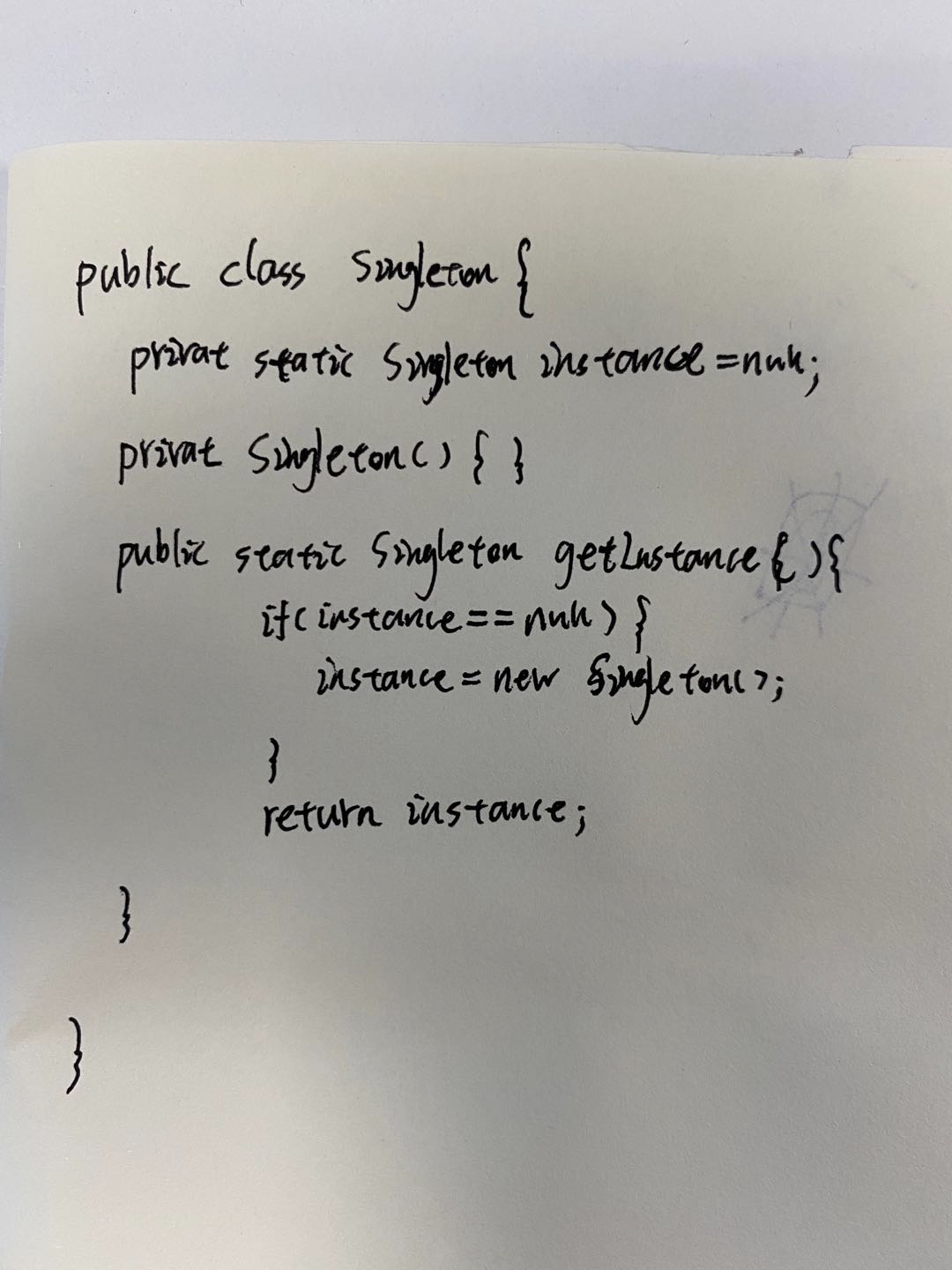
2、组合设计模式打印窗口组件
类图设计
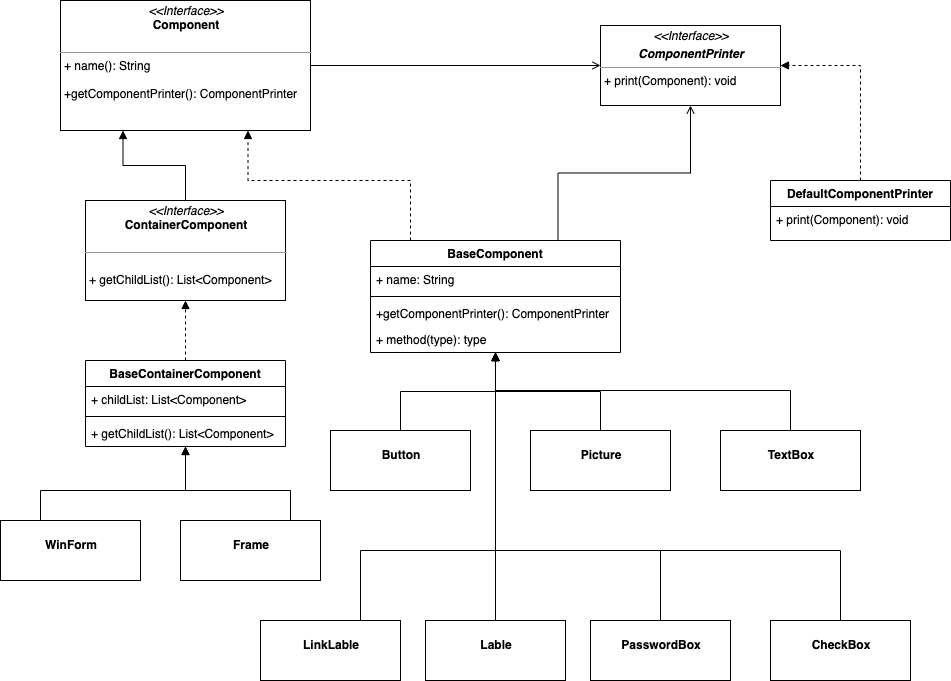
示例代码
/** * 组件接口 */public interface Component { String name(); ComponentPrinter getComponentPrinter();}/** * 容器组件:具有子元素的组件 */public interface ContainerComponent extends Component { List<Component> getChildList(); void addChildComponent(Component child);}//组件打印器 接口public interface ComponentPrinter{ void print(Component component); }//默认打印器public class DefaultComponentPrinter implements ComponentPrinter { @Override public void print(Component component) { //打印component的name doPrint(component.name()); //如果是容器组件,遍历子元素打印 if (component instanceof ContainerComponent) { List<Component> childList = ((ContainerComponent) component).getChildList(); if (childList != null) { for (Component item : childList) { //调用组件自身打印器 打印 item.getComponentPrinter().print(item); } } } } /** * 执行打印 */ private void doPrint(String content) { System.out.println("print " + content); }}/** * 组件 抽象实现类 */public abstract class BaseComponent implements Component { protected String name; protected ComponentPrinter componentPrinter; public BaseComponent(String name) { this.name = name; this.componentPrinter = new DefaultComponentPrinter(); } public BaseComponent() { } @Override public String name() { return this.getClass().getSimpleName() + "(" + name + ")"; } @Override public ComponentPrinter getComponentPrinter() { return componentPrinter; }}/** * 容器组件 抽象实现类 */public abstract class BaseContainerComponent extends BaseComponent implements ContainerComponent { protected List<Component> childList; public BaseContainerComponent(String name) { super(name); this.childList = new ArrayList<>(); } @Override public List<Component> getChildList() { return childList; } @Override public void addChildComponent(Component child) { if (childList == null) { childList = new ArrayList<>(); } childList.add(child); }}/** * 窗口组件-is a 容器组件 */public class WinForm extends BaseContainerComponent { public WinForm(String name) { super(name); }}/** * 按钮 是一个组件 */public class Button extends BaseComponent { public Button(String name) { super(name); }}
以上是组件的实现。定义Component接口代表基础组件,定义ContainerComponent接口代表 容器组件,可以拥有子组件列表。
public static void main(String[] args) { ContainerComponent windowForm = new WinForm("WINDOW窗口"); Component picture = new Picture("LOGO图片"); windowForm.addChildComponent(picture); //windowForm添加子组件 Component loginButton = new Picture("登录"); windowForm.addChildComponent(loginButton); ContainerComponent frame = new Frame("FRAME1"); //windowForm 添加 Frame 容器组件 windowForm.addChildComponent(frame); //...省略其他代码 Component textBook2 = new TextBox("记住用户名"); //Frame添加子组件 frame.addChildComponent(textBook2); //执行打印 windowForm.getComponentPrinter().print(windowForm); }
输出结果:
print WinForm(WINDOW窗口)print Picture(LOGO图片)print Picture(登录)print Picture(注册)print Frame(FRAME1)print Label(用户名)print TextBox(文本框)print Label(密码)print PasswordBox(密码框)print CheckBox(复选框)print TextBox(记住用户名)print LinkLable(忘记密码)
划线
评论
复制
发布于: 2020 年 09 月 28 日 阅读数: 18
道长
关注
还未添加个人签名 2018.03.11 加入
还未添加个人简介
评论