第三周作业
发布于: 2020 年 06 月 22 日
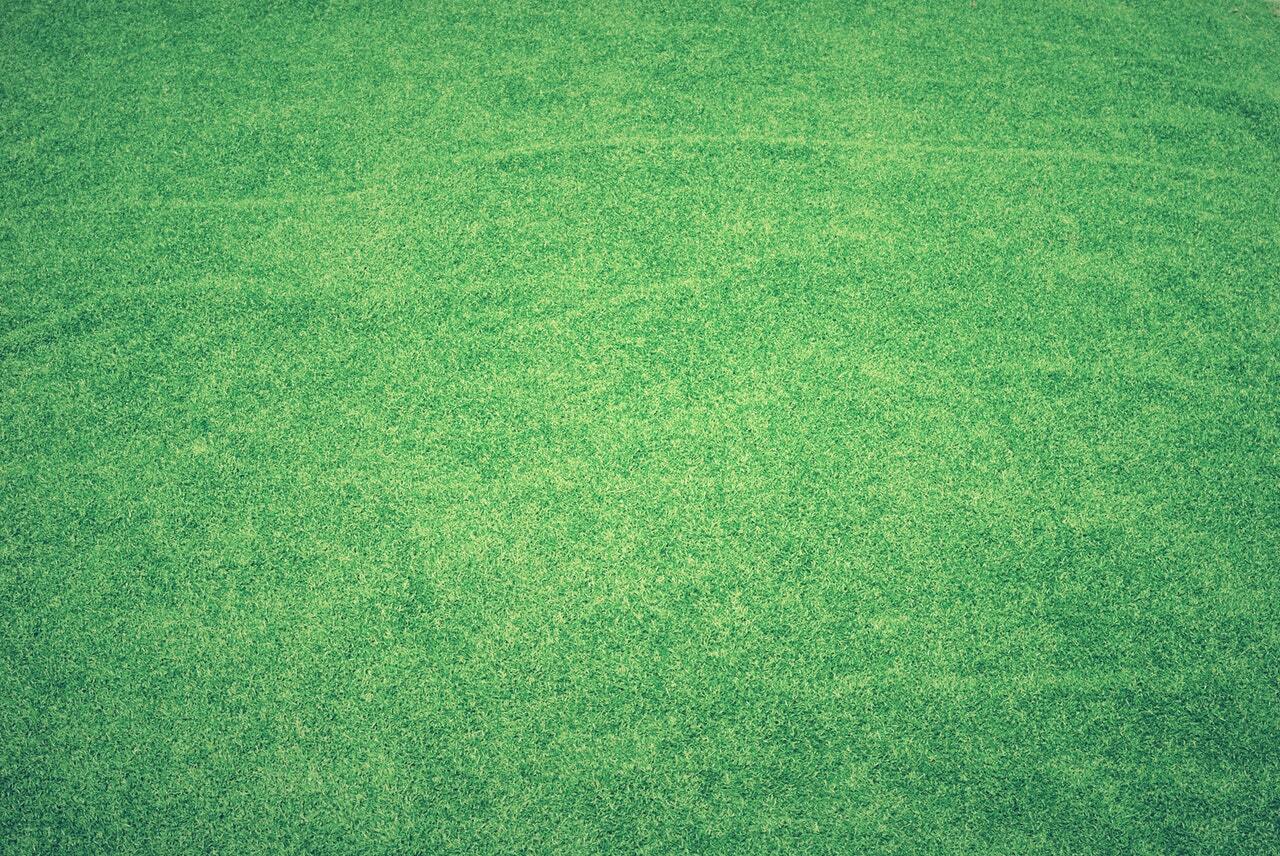
作业一:
请在草稿纸上手写一个单例模式的实现代码,拍照提交作业。
单例模式之饿汉模式
单例模式之懒汉模式-方法锁
单例模式之懒汉模式-双重检查锁
单例模式之懒汉模式-静态内部类
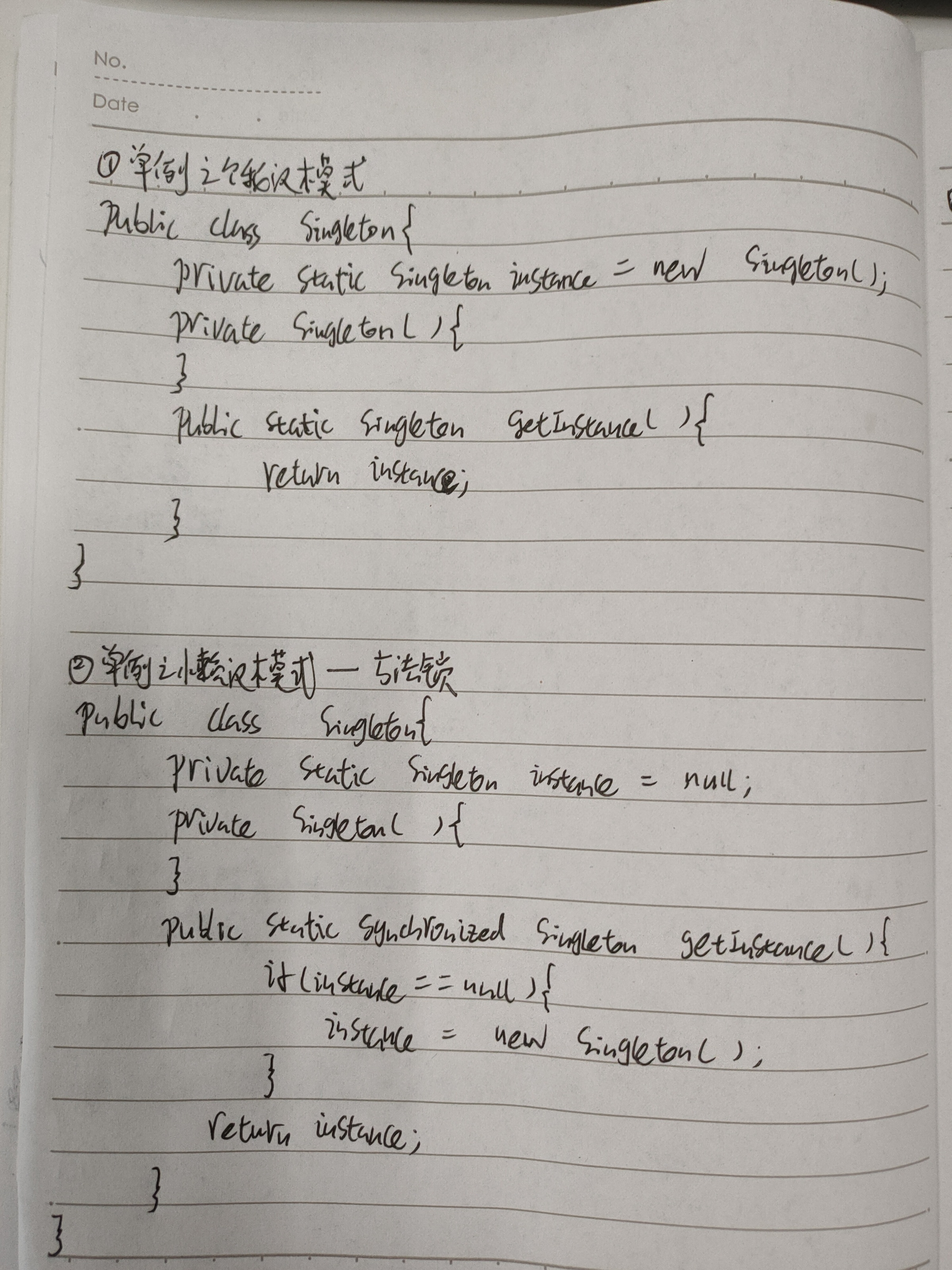
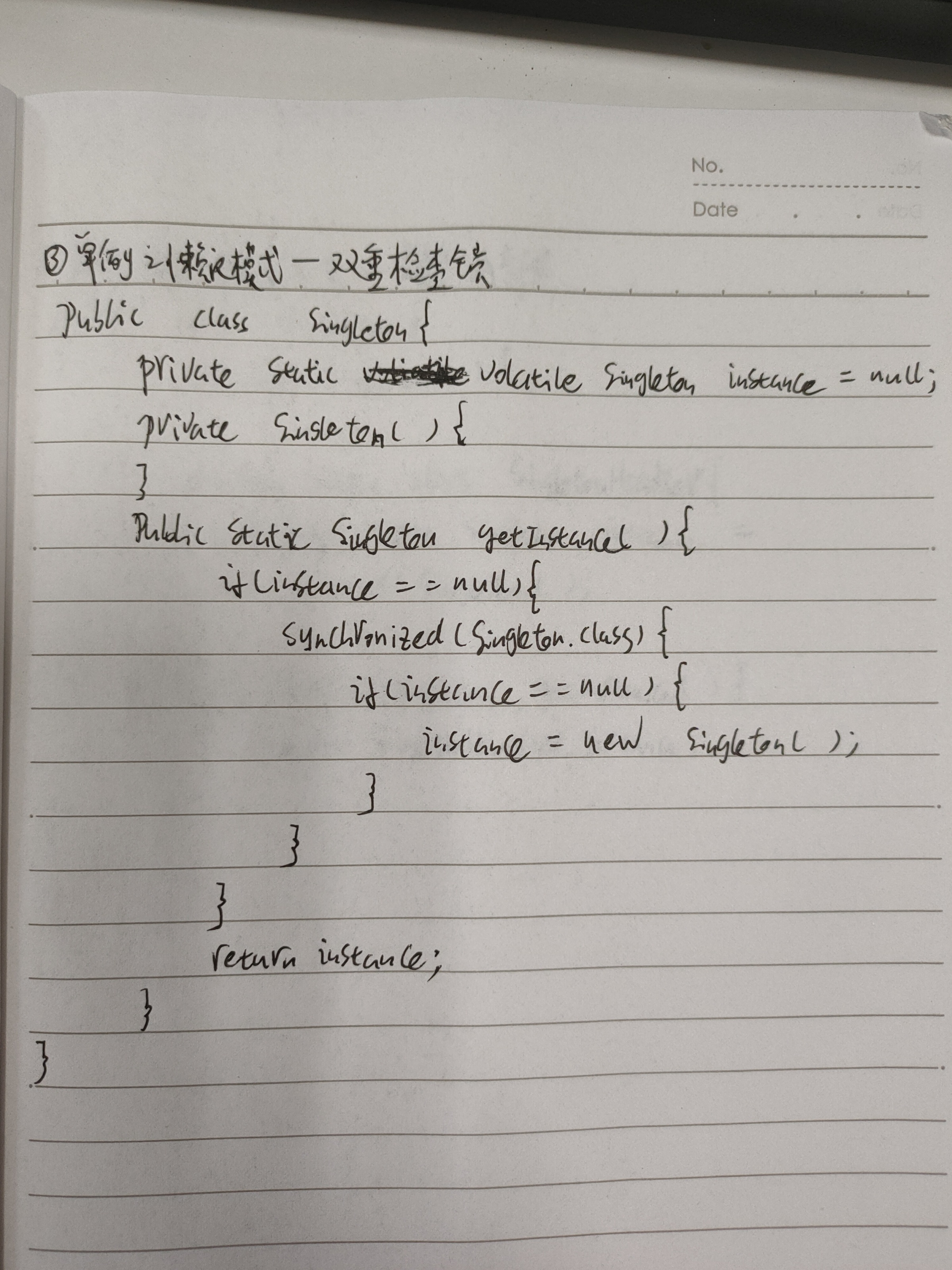
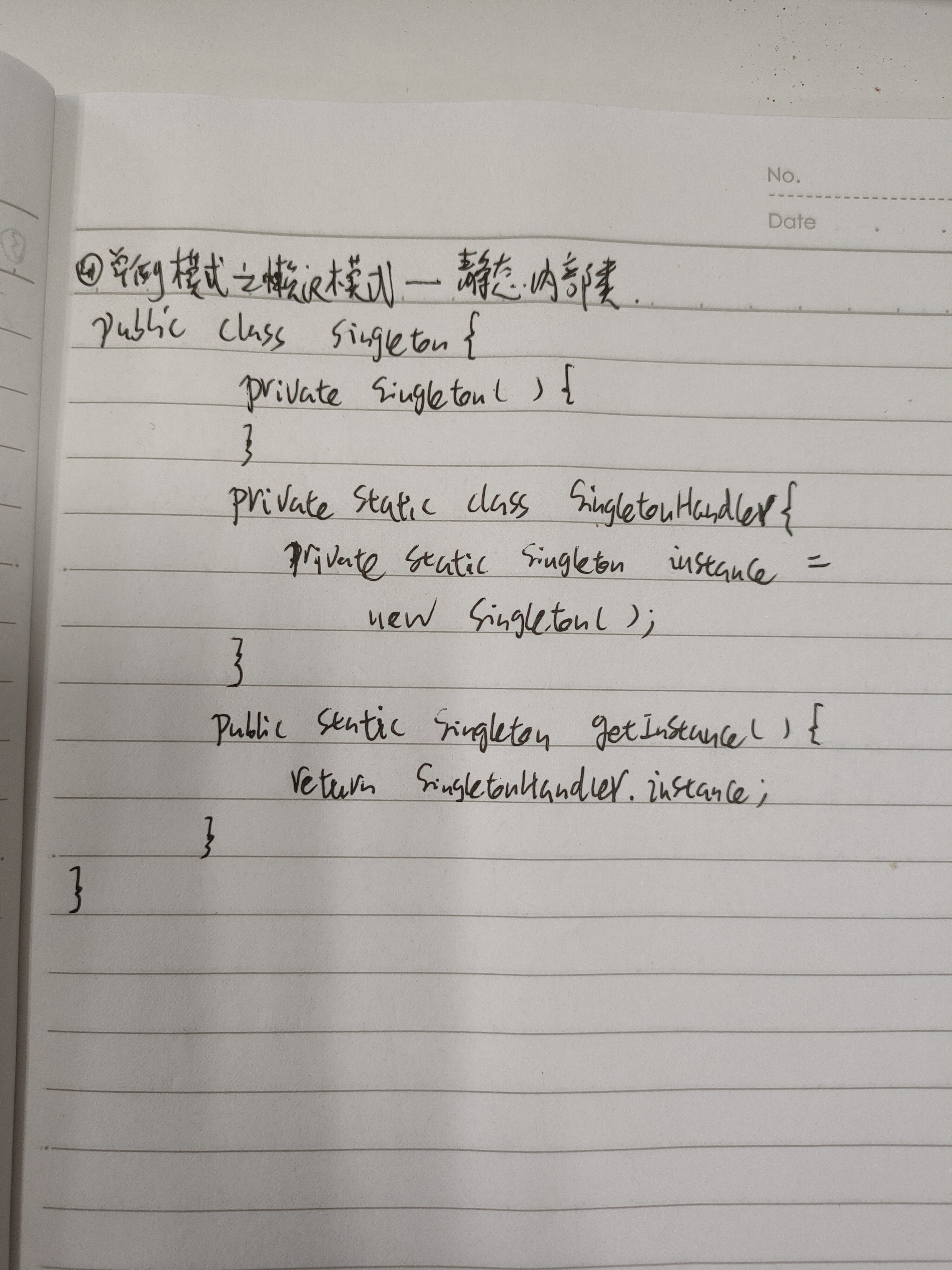
饿汉模式简单好理解,类被jvm加载后就创建了单例的对象,缺点就是有可能这个单例对象一直没有被使用,但是已经在堆内存中了,造成了资源的浪费。
懒汉模式的好处就只有用到单例对象的时候才会去创建对象,当然在多线程的场景下,就会带来线程安全的问题。在方法上加互斥锁可以解决线程安全的问题,但是锁的粒度太粗,锁的范围应该是创建对象的那部分,如果已经存在创建好单例的对象,这个时候去获取对象,是不应该有锁的。
双重检查锁,细化了互斥锁的粒度,只有在创建对象的代码块上了锁,解决了上面提到的两个问题(延迟创建对象,获取对象无锁),但是代码复杂度较高。
静态内部类是使用了jvm类加载机制去创建单例对象,满足上面提到的两个问题,只有在调用Singleton.getInstance()方法jvm才会去加载SingletonHandler类,同时jvm会保证线程安全,不会创建出多个单例对象,一旦初始化完成完成,以后再获取单例对象就是无锁的了,是比较好的解决方案。
作业二:
请用组合设计模式编写程序,打印输出图 1 的窗口,窗口组件的树结构如图 2 所示,打印输出示例参考图 3。
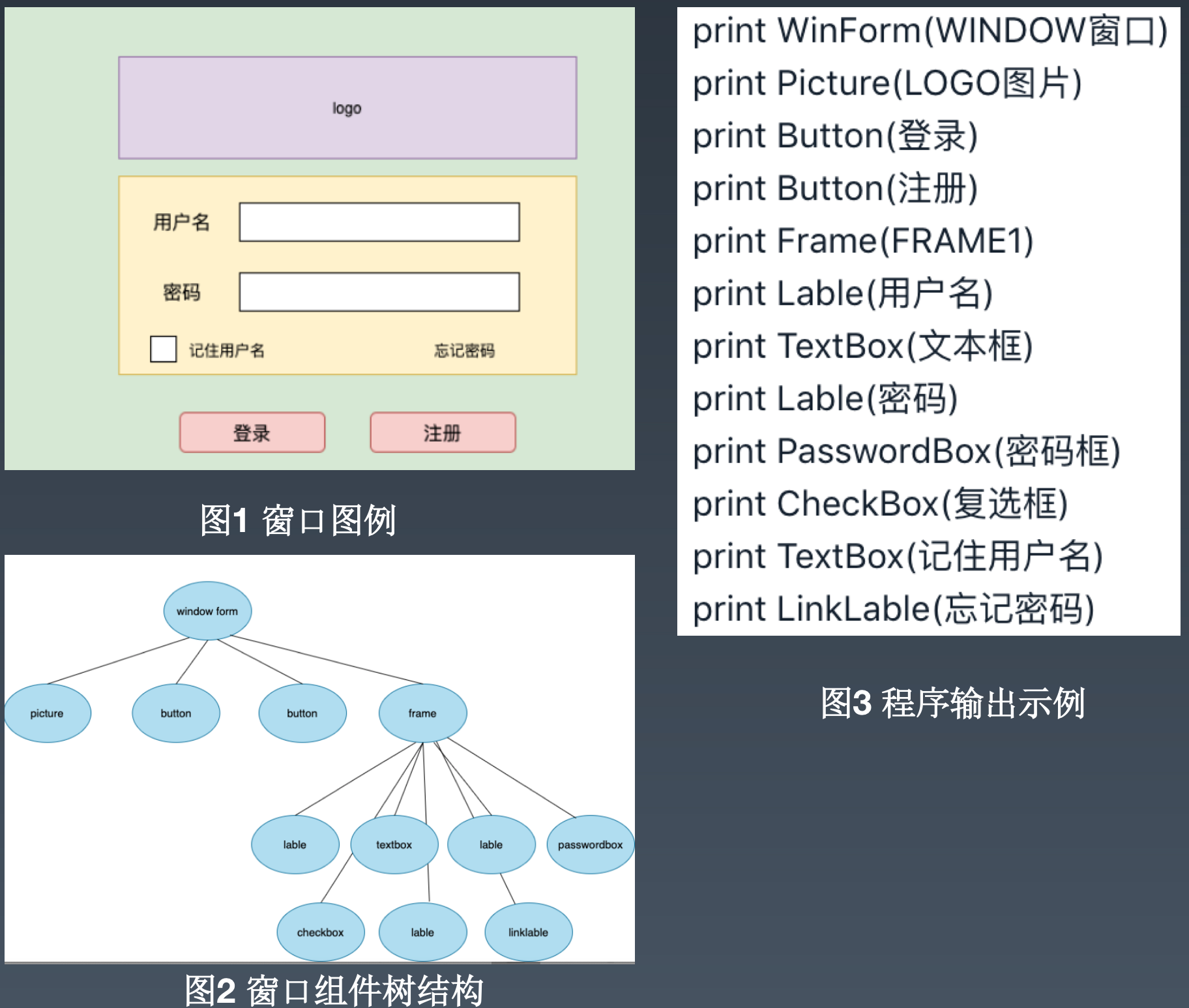
组合设计模式实现
类图:
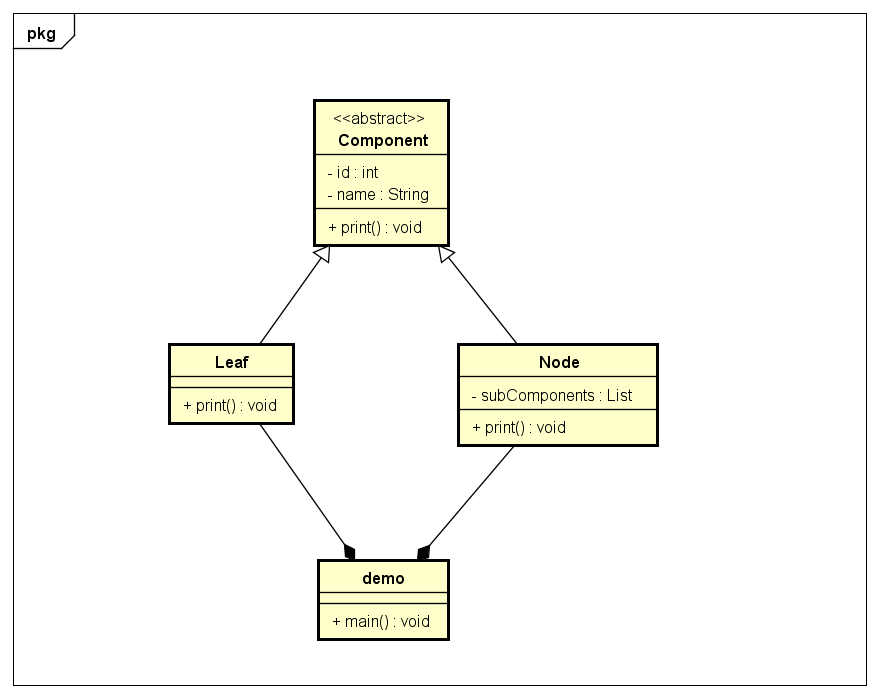
代码如下:
public abstract class Component { protected int x; protected int y; protected int width; protected int height; protected int id; protected String name; public Component(int id, String name){ this.id = id; this.name = name; } /** * 打印组件 */ public abstract void print();}
public class Leaf extends Component{ public Leaf(int id, String name){ super(id, name); } @Override public void print() { System.out.println(String.format("print %s", name)); }}
public class Node extends Component{ private List<Component> subComponents = new ArrayList<>(); public Node(int id, String name){ super(id, name); } @Override public void print() { System.out.println(String.format("print %s", name)); for(Component component : subComponents){ component.print(); } } public void addSubComponent(Component component) { subComponents.add(component); }}
public class Demo { public static void main(String[] args) { Node rootNode = new Node(0, "WinForm(WINDOW窗口)"); Leaf logoPicture = new Leaf(1, "Picture(LOGO图片)"); Node frame = new Node(2, "Frame(FRAME1)"); Leaf usernameLabel = new Leaf(21, "Label(用户名)"); Leaf textBox = new Leaf(22, "TextBox(文本框)"); Leaf passwordLabel = new Leaf(23, "Label(密码)"); Leaf passwordBox = new Leaf(24, "PasswordBox(密码框)"); Leaf checkBox = new Leaf(25, "CheckBox(复选框)"); Leaf rememberLabel = new Leaf(26, "TextBox(记住用户名)"); Leaf linkLabel = new Leaf(27, "LinkLabel(忘记密码)"); frame.addSubComponent(usernameLabel); frame.addSubComponent(textBox); frame.addSubComponent(passwordLabel); frame.addSubComponent(passwordBox); frame.addSubComponent(checkBox); frame.addSubComponent(rememberLabel); frame.addSubComponent(linkLabel); Leaf loginButton = new Leaf(3, "Button(登录)"); Leaf registerButton = new Leaf(4, "Button(注册)"); rootNode.addSubComponent(logoPicture); rootNode.addSubComponent(loginButton); rootNode.addSubComponent(registerButton); rootNode.addSubComponent(frame); rootNode.print(); }}
递归方法实现
public class RecursiveComponent { private int x; private int y; private int width; private int height; private int id; private String name; private boolean isNode; private List<RecursiveComponent> subComponents = new ArrayList<>(); public RecursiveComponent(int id, String name, boolean isNode){ this.id = id; this.name = name; this.isNode = isNode; } public void addSubComponent(RecursiveComponent component) { subComponents.add(component); } public void print() { System.out.println(String.format("print %s", name)); if(isNode){ for(RecursiveComponent component : subComponents){ component.print(); } } }}
public class RecursiveDemo { public static void main(String[] args) { RecursiveComponent rootNode = new RecursiveComponent(0, "WinForm(WINDOW窗口)", true); RecursiveComponent logoPicture = new RecursiveComponent(1, "Picture(LOGO图片)", false); RecursiveComponent frame = new RecursiveComponent(2, "Frame(FRAME1)", true); RecursiveComponent usernameLabel = new RecursiveComponent(21, "Label(用户名)", false); RecursiveComponent textBox = new RecursiveComponent(22, "TextBox(文本框)", false); RecursiveComponent passwordLabel = new RecursiveComponent(23, "Label(密码)", false); RecursiveComponent passwordBox = new RecursiveComponent(24, "PasswordBox(密码框)", false); RecursiveComponent checkBox = new RecursiveComponent(25, "CheckBox(复选框)", false); RecursiveComponent rememberLabel = new RecursiveComponent(26, "TextBox(记住用户名)", false); RecursiveComponent linkLabel = new RecursiveComponent(27, "LinkLabel(忘记密码)", false); frame.addSubComponent(usernameLabel); frame.addSubComponent(textBox); frame.addSubComponent(passwordLabel); frame.addSubComponent(passwordBox); frame.addSubComponent(checkBox); frame.addSubComponent(rememberLabel); frame.addSubComponent(linkLabel); RecursiveComponent loginButton = new RecursiveComponent(3, "Button(登录)", false); RecursiveComponent registerButton = new RecursiveComponent(4, "Button(注册)", false); rootNode.addSubComponent(logoPicture); rootNode.addSubComponent(loginButton); rootNode.addSubComponent(registerButton); rootNode.addSubComponent(frame); rootNode.print(); }}
总结:
相对递归方法,组合设计模式写出来的代码架构清晰,可读性更好,扩展性更强。
划线
评论
复制
发布于: 2020 年 06 月 22 日阅读数: 70
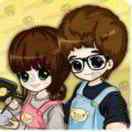
changtai
关注
还未添加个人签名 2018.04.30 加入
还未添加个人简介
评论